Display User Location
This section of the iOS Maps SDK provides developers with the necessary tools and guidelines to incorporate user location functionality into their applications. This functionality allows users to see their current location on the map, providing a more personalized and interactive experience.
Permissions
This section explains the importance of obtaining the necessary location permissions from the user. It provides information on how to request location permissions and handle user responses. Ensuring that the appropriate permissions are obtained is crucial for accessing and displaying the user's location accurately and securely.
Add the following to the info.plist
Replace YOUR_DESCRIPTION with a short description of the location information your application uses.
Permission requests are automatically triggered by the Maps SDK when obtaining location information
Location provider/Component
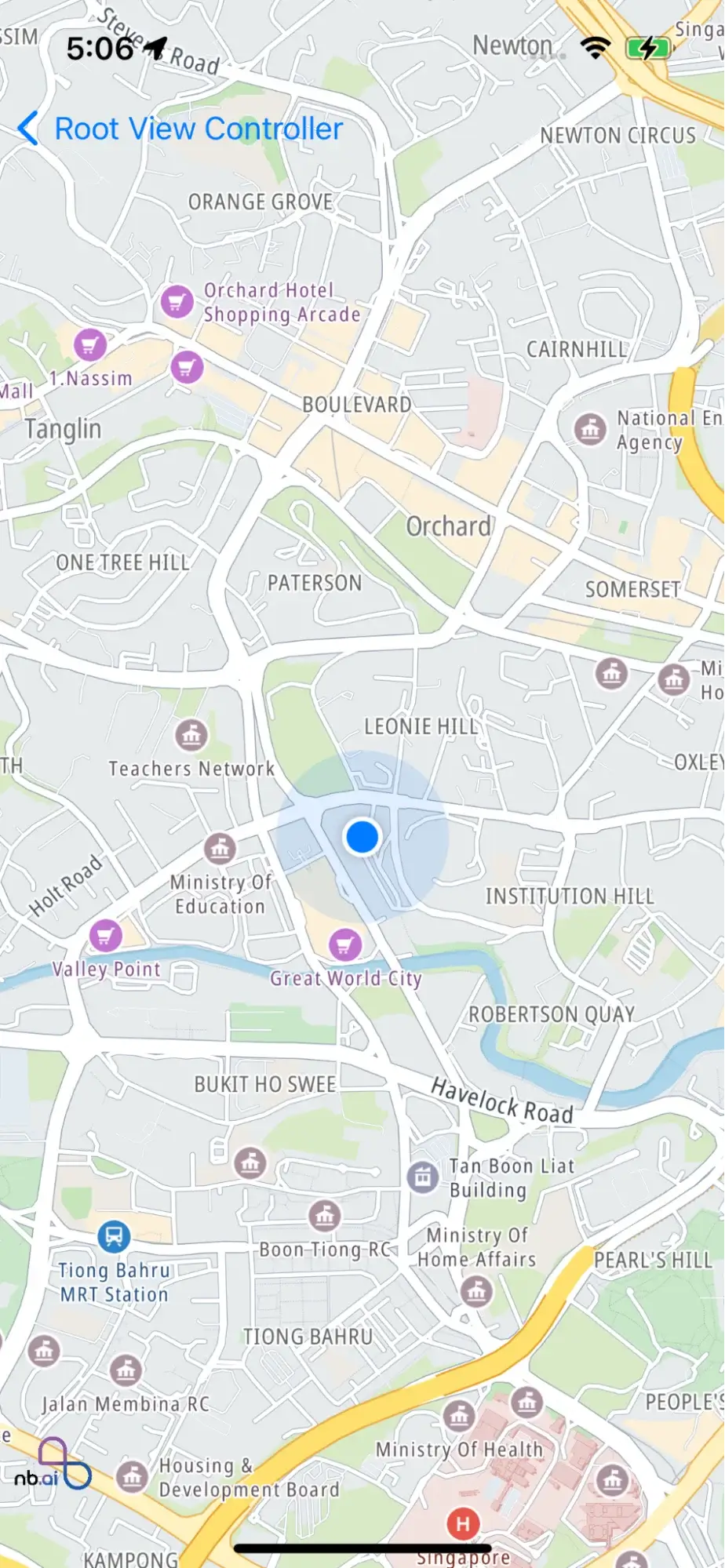
You can use the following code to display the current location on the map. mapView.showsUserLocation = true
You can also use your own location component. Here is an example of MapView
using a custom Location component.
-
Create custom
MapLocationManager
-
Use custom location manager
Location Puck
The location puck refers to the graphical representation of the user's current location on the map. This subsection discusses how to customize and style the location puck to match the app's design and provide a clear visual indication of the user's position on the map. Developers can learn how to adjust the size, color, and shape of the location puck to meet their application's requirements.
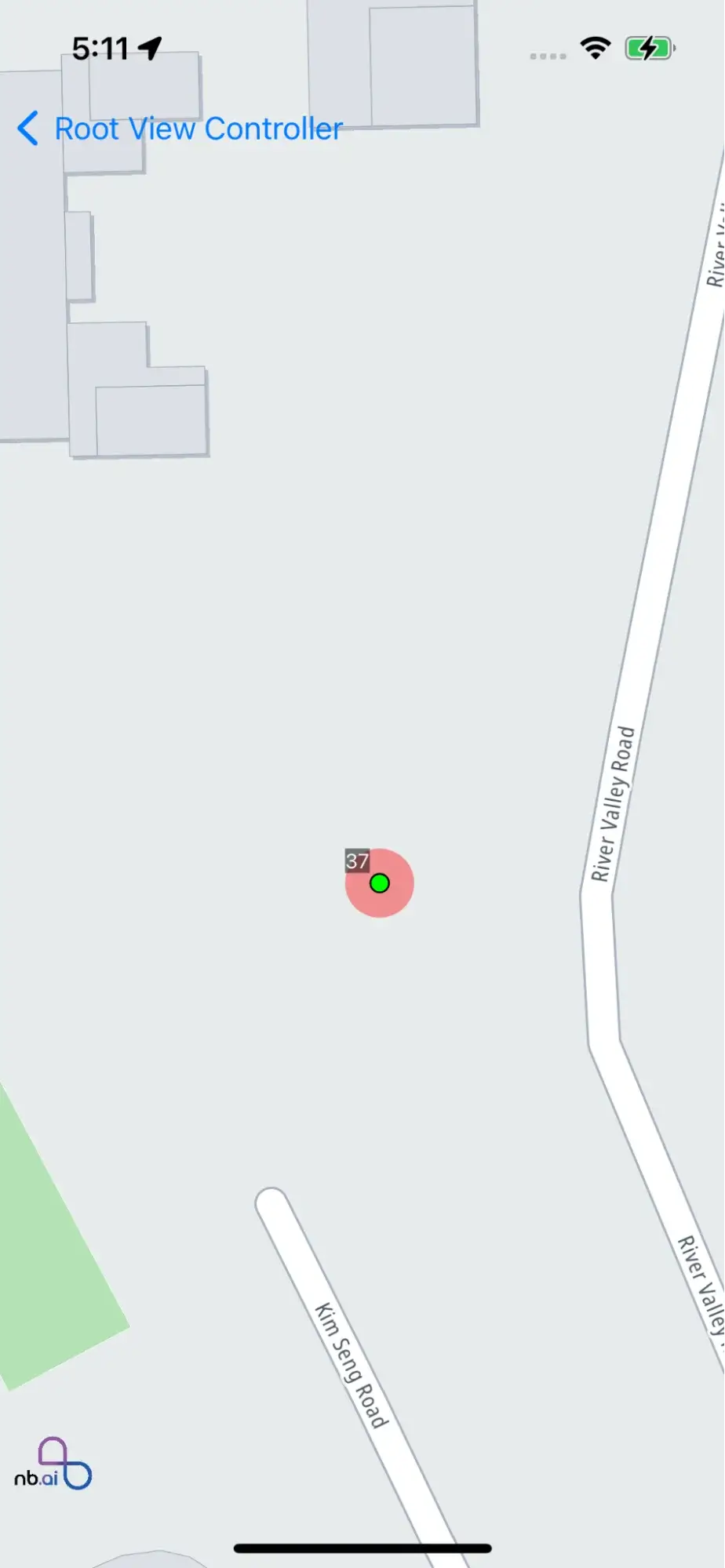
Code example: show user location on the map and customise puck view
Location tracking mode
This subsection focuses on different location tracking modes available in the iOS Maps SDK. It explains the concept of location tracking modes, such as follow
, follow with course
, and follow with heading
. Developers can understand how each tracking mode works and choose the appropriate one based on their application's needs. These modes enable the map to dynamically adjust and center on the user's location as they move, providing a smooth and intuitive user experience.
The provided code example demonstrates how to show the user's location on a map and change the tracking mode using the iOS Maps SDK.
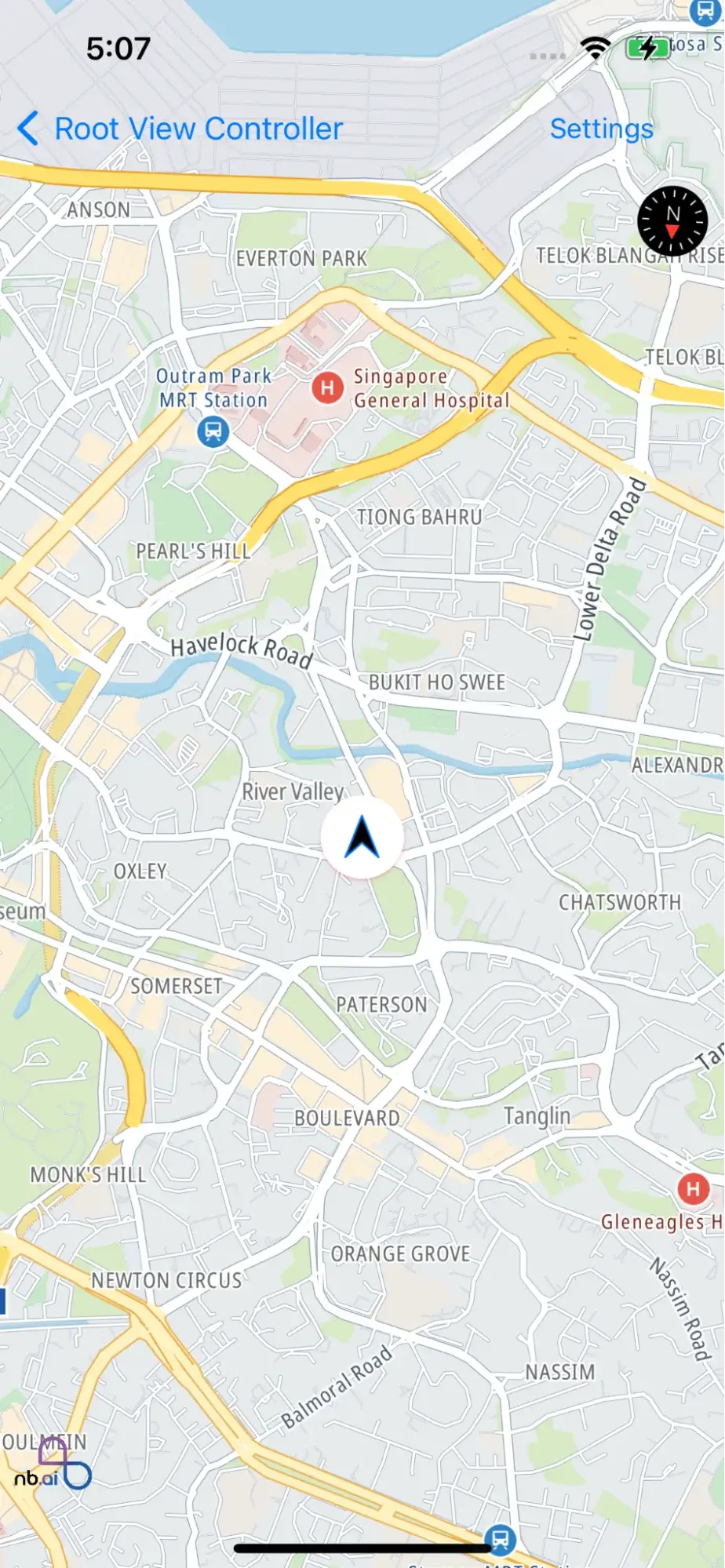
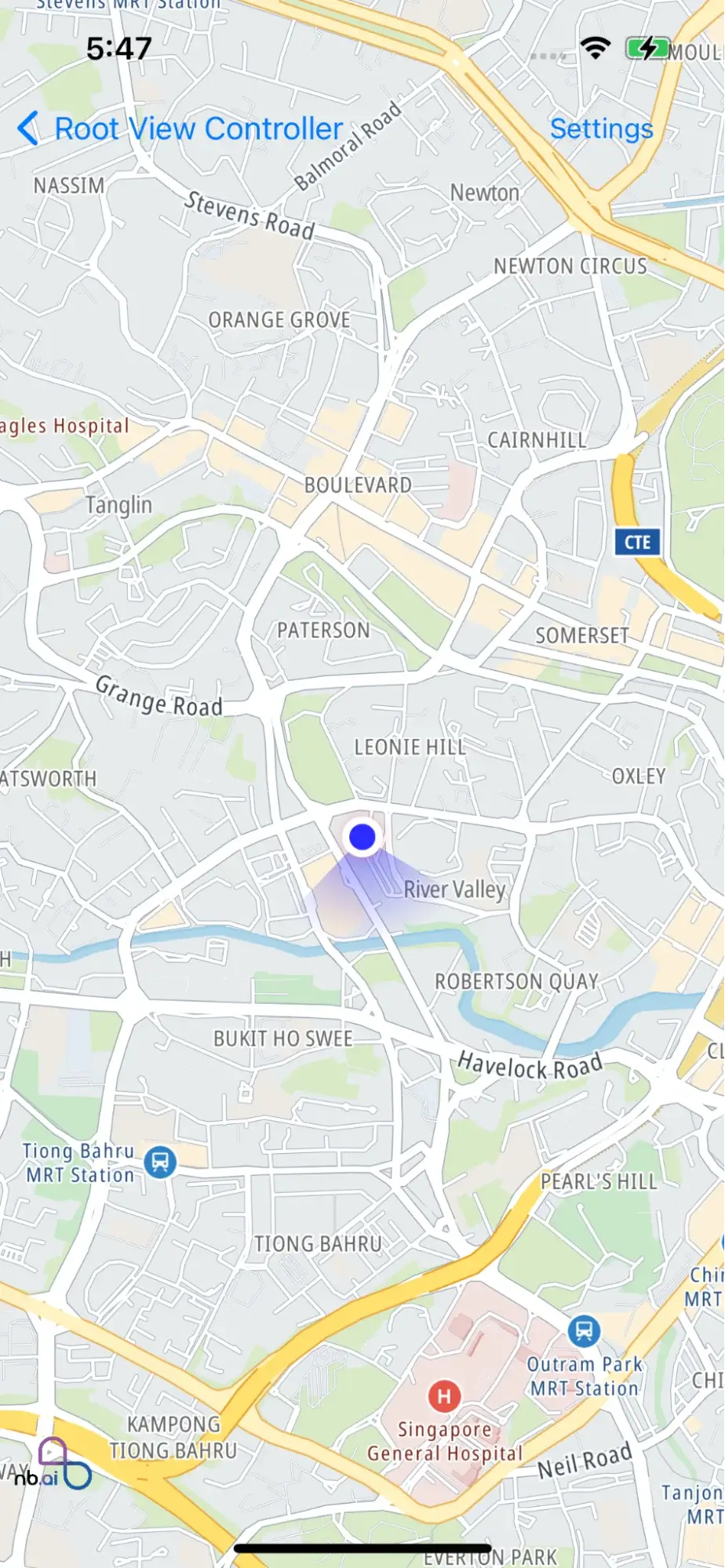
Let's break down the code:
The code begins with the import statements for the required frameworks, UIKit
and Nbmap
.
Enum Declaration:
- The
LocationType
enum defines different types of location settings, such as hiding the puck view, updating to follow the user's location, updating to follow with a heading, updating to follow with a course, and getting the user's location.
Class Declaration:
-
The
LocationStyleViewController
class is a subclass of UIViewController and serves as the main view controller for the location functionality. -
It declares a property
nbMapView
of type NGLMapView to display the map view and handles its setup and removal. -
It also declares a property
button
of type UIButton to show a settings button on the navigation bar. -
The class includes an array
typeList
that stores the different LocationType options. -
The
viewDidLoad()
method initializes the nbMapView and sets its delegate. It also sets up the settings button on the navigation bar. -
The
showSettings()
method is called when the settings button is tapped. It presents a table view controller to display location settings options. -
The
performSettings(type:)
method is called when a location setting is selected. It performs the corresponding action based on the selected LocationType.
Extensions:
-
The
LocationStyleViewController
extension implements theNGLMapViewDelegate
protocol, providing necessary delegate methods for the map view. -
The
mapView(_:didFinishLoading:)
method is called when the map finishes loading. It sets the initial camera position. -
Other delegate methods, such as
mapViewWillStartLocatingUser
,mapViewDidStopLocatingUser
, andmapView(_:didChange:animated:)
, are implemented but are currently empty. They can be used to handle various events related to user location tracking. -
The
mapView(styleForDefaultUserLocationAnnotationView:)
method customizes the appearance of the user location annotation view by setting properties such as puckFillColor, puckShadowColor, and haloFillColor. -
The
mapView(_:didUpdate:)
method is called when the user's location is updated. Currently, it is empty and can be used to perform actions based on the user's updated location. -
Other delegate methods, such as
mapView(_:didFailToLocateUserWithError:)
andmapView(_:didChangeLocationManagerAuthorization:)
, are implemented but are currently empty.
Additional Extensions:
-
The
LocationStyleViewController
extension implements the UITableViewDelegate and UITableViewDataSource protocols to handle the settings table view. -
The methods in these extensions populate the table view with the available location settings options and handle selection.
-
The
dismissSettings(type:)
method is called when a location setting is selected, and it dismisses the settings view controller and performs the corresponding action.
Overall, the code example provides a view controller that displays a map view, handles user location tracking, and allows the user to change the location tracking mode based on different settings.