Center Camera In Bounds
This example shows how to Center Camera in Bounds
-
Create polygon with custom bound area
-
Center Camera to given bounds and padding
-
mMap.animateCamera(CameraUpdateFactory.newLatLngBounds(bounds, 150))
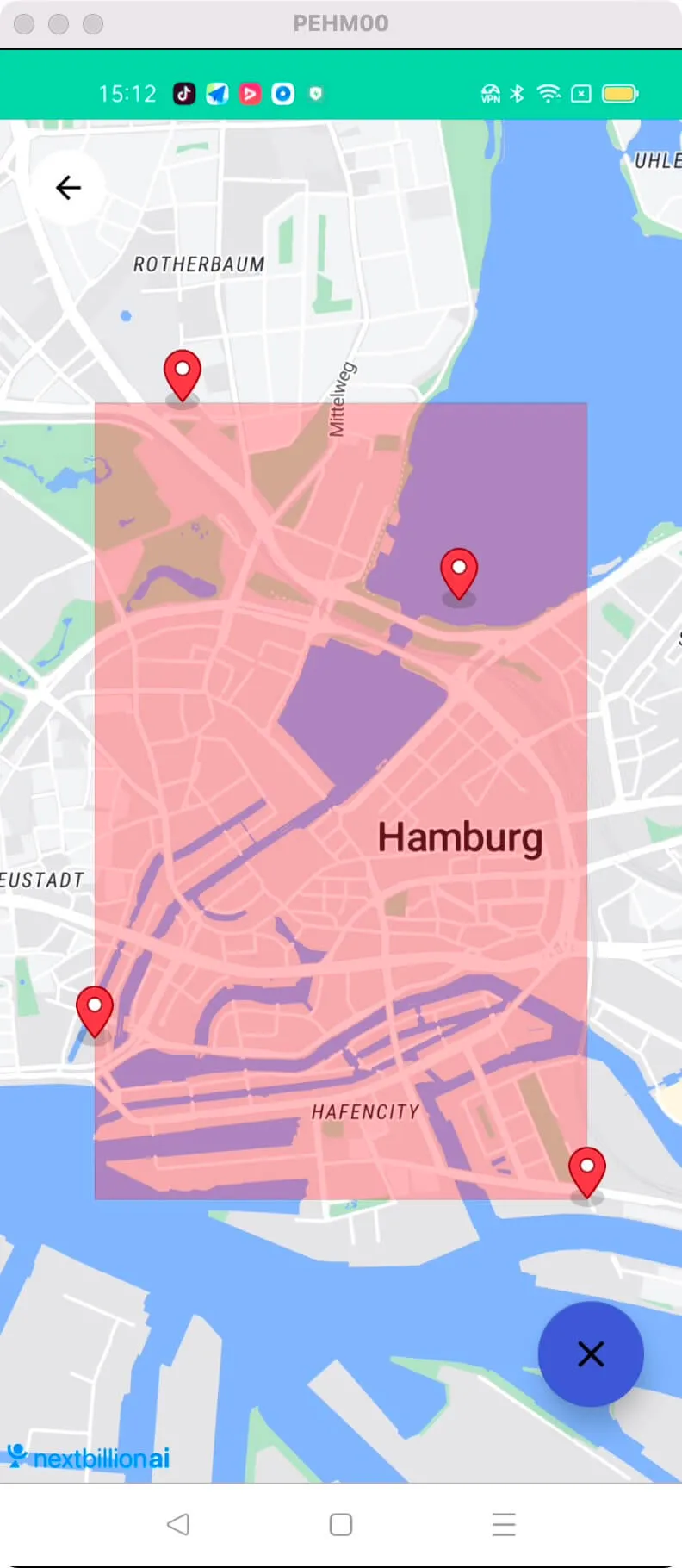
For all code examples, refer to Android Maps SDK Code Examples
activity_bounds.xml view source
BoundsActivity view source
The given code is an Android activity that allows users to create a polygon by selecting points on a map. It also provides the functionality to remove the created polygon.
Initializing the MapView:
-
The MapView is initialized in the onCreate method using binding.mapView.onCreate(savedInstanceState).
-
The map is obtained asynchronously using binding.mapView.getMapAsync and the onMapSync method is called.
Creating a Polygon with Custom Bound Area:
-
The onMapSync method sets up the NextbillionMap and adds a map long click listener.
-
When a long click event occurs, the checkBounds method is called with the clicked LatLng coordinate.
-
The clicked LatLng is added to the boundsPoints list, and a marker is placed at that location on the map.
-
If there are at least 4 points in the boundsPoints list, a polygon is created with the bounds using LatLngBounds.Builder().includes(boundsPoints).build().
-
The existing bounds area is removed from the map using mMap.removePolygon(it.polygon).
-
A new PolygonOptions object is created and added to the map with the appropriate points and styling.
-
The camera is animated to center on the created bounds using mMap.animateCamera(CameraUpdateFactory.newLatLngBounds(bounds, 150)).
Centering Camera to Given Bounds and Padding:
-
The mMap.setLatLngBoundsForCameraTarget(bounds) method is used to set the bounds for the camera movement.
-
The camera is animated to center on the created bounds with a padding of 150 pixels.
Removing Bounds:
-
The removeBounds method is called when the "removeBounds" button is clicked.
-
It clears the map, clears the boundsPoints list, sets boundsArea to null, and hides the "removeBounds" button. Lifecycle Methods:
The code also includes various lifecycle methods (onStart, onResume, onPause, onStop, onSaveInstanceState, onDestroy, onLowMemory) that should be implemented when using the MapView to properly manage its lifecycle and handle configuration changes.
Note: The code uses the Nextbillion Maps SDK, which provides map-related functionalities. It also utilizes the LatLng class to represent geographical coordinates and the LatLngBounds class to define a rectangular area on the map.