Basic Location Tracking
This example shows how to Track the Current Location Manually
- Location Permissions Handling
- Tracking current location automatically when Map ready
- Add CameraTracking Change Listener to update tracking status
- Click Location Tracking Button to track the current location
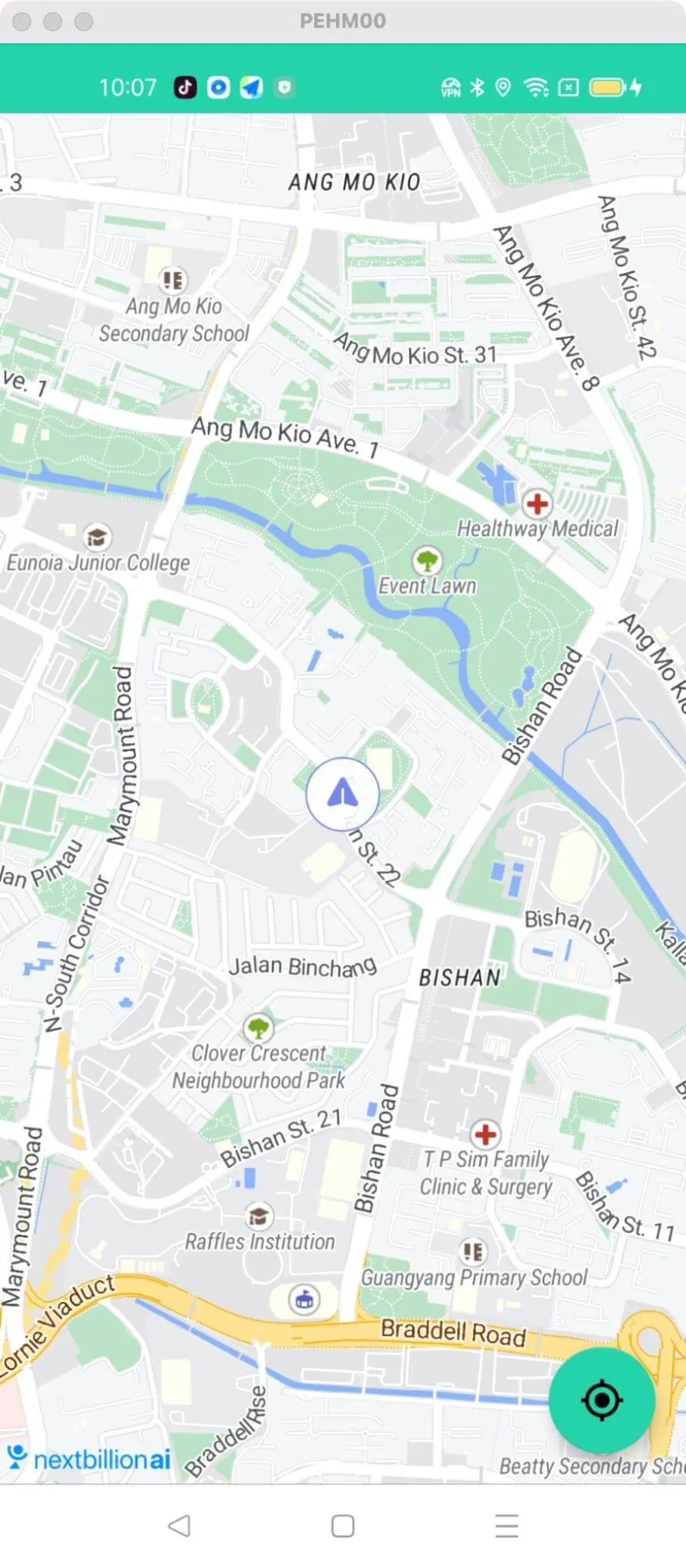
For all code examples, refer to Android Maps SDK Code Examples
activity_basic_location_tracking.xml view source
BasicLocationTrackingActivity view source
This code example demonstrates basic location-tracking functionality using the Nextbillion map library. It handles location permissions, tracks the current location automatically when the map is ready, adds a camera tracking change listener to update the tracking status, and allows the user to click a button to track their current location.
Location Permissions Handling:
- The code checks if location permissions are granted using PermissionsManager.areLocationPermissionsGranted(this).
- If permissions are granted, the map is asynchronously loaded using mapView.getMapAsync(this).
- If permissions are not granted, a PermissionsManager instance is created and used to request location permissions. The result of the permission request is handled in the onPermissionResult method.
Tracking Current Location Automatically when Map Ready:
- The onMapReady method is called when the map is ready.
- The nextbillionMap instance is assigned.
- A CameraPosition is created to set the initial camera position of the map.
- The map style is set using a Style.Builder and the activateLocationComponent method are called to activate the location component.
Add CameraTracking Change Listener to Update Tracking Status:
- The activateLocationComponent method is called when the location component is activated.
- The OnCameraTrackingChangedListener is added to the location component to listen for changes in camera tracking status.
- When tracking is dismissed, the tracking button's image is updated.
- The addOnLocationClickListener and addOnLocationLongClickListener methods are used to listen for location clicks and long clicks.
Click Location Tracking Button to Track Current Location:
- The trackLocation button's click listener is implemented.
- When clicked, the button image is updated, and the trackLocation method is called.
- In the trackLocation method, the location component is retrieved from the nextbillionMap and configured.
- The location component's style is updated to enable pulsing.
- The location component is enabled, and the CameraMode is set to TRACKING.
Additional notes:
- The code includes lifecycle methods (onStart, onResume, onPause, onStop, onSaveInstanceState, onDestroy, onLowMemory) to manage the lifecycle of the MapView.
- The code uses Nextbillion map library classes and methods for map-related operations.
- The code includes resource IDs for icons and handles their display and click events.
- The code demonstrates how to handle location permissions using the PermissionsManager class.