Dispatch Optimized Routes to Geotab
To dispatch the generated routes to the MyGeoTab Web Application, follow these detailed steps. This process involves constructing a POST request from the routes generated by NextBillion.ai’s Route Optimization API and sending it to Geotab.
Step 1: Extract Relevant Data from NextBillion.ai Result
First, retrieve the optimization result data, including the routes, from the NextBillion.ai API response.
Step 2: Define Helper Function to Convert Distance to Miles
Create a helper function to convert distances from meters to miles.
Step 3: Get Zone IDs from Dropdowns
Retrieve the zone IDs for the start and end locations from dropdown elements.
Step 4: Construct the POST Request Body
Build the request body for the POST request to dispatch routes to Geotab.
Step 5: Convert Request Body to JSON String
Convert the constructed request body to a JSON string for the POST request.
Step 6: Print and Store the Request Body
Print the request body to the console and store it in local storage for further use.
Step 7: Trigger the POST Request to Geotab
send_to_geotab.trigger
is a function that sends the POST request, use it to dispatch the route to Geotab. Handle success and failure responses appropriately.
Step 8: Send POST Dispatch Request
Here’s an example of how the POST request will look using curl:
API Response
By following these steps, you can successfully dispatch the generated routes to the MyGeoTab Web Application.
The following images showcases the routes dispatched to your MyGeotab dashboard for each driver.
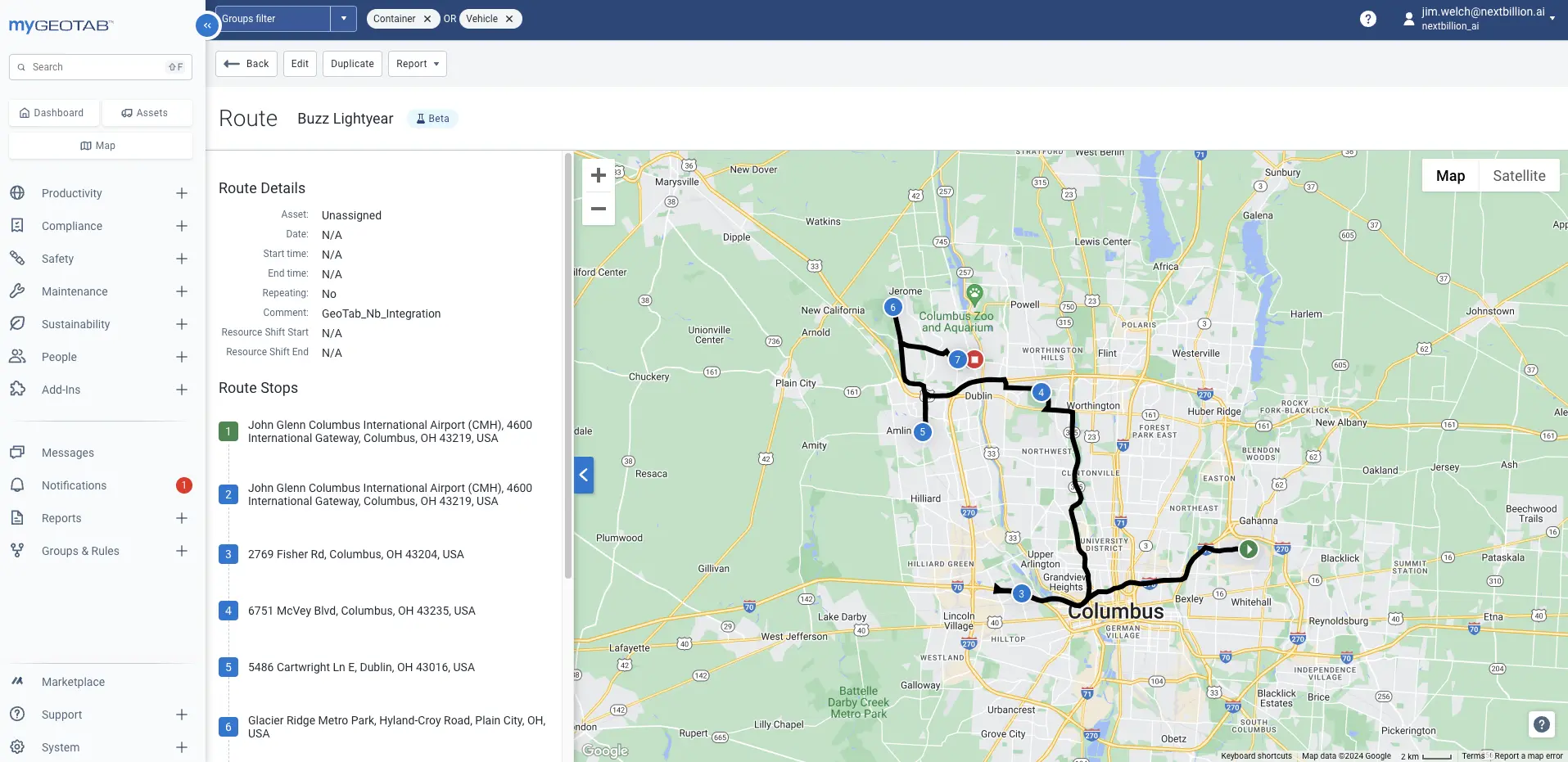
Integrating NextBillion.ai’s Route Optimization API with the GeoTab Web streamlines the process of generating and dispatching optimized routes. This integration enhances operational efficiency by leveraging advanced route optimization capabilities, thus ensuring that drivers follow the most efficient paths for their deliveries.