Map Display and Navigation
Generally, a navigation application comprises two pages incorporating a map view. We will refer to this setup as a standalone navigation view.
Users can designate the destinations or waypoints on the initial page, which they intend to traverse. Simultaneously, a route is obtained and displayed within the map view. Upon selecting "start navigation," the application transitions to the second page, providing a comprehensive navigation experience.
In certain scenarios, product designers may prefer to consolidate waypoint selection and navigation initiation onto a single page. We will refer to this alternative as the embedded navigation view. While this approach is discussed in other sections, the present section focuses on the conventional case (i.e., the standalone navigation view).
To embark on your first navigation experience, construct a page for plotting waypoints and execute an HTTP request to acquire a route. Subsequently, access the built-in navigation page by invoking the SDK API.
First Page
Integrate a map view
Normally, we only initialize NavigationMapView once, so we call it in viewDidLoad()
Get the user’s current location
To obtain users’ geographic location, we are using Core Location Service via instances of CLLocationManager, in our SDK we can leverage NavigationLocationManager to determine users’ location.
On the other hand, you can also implement CLLocationManager’s delegation to manage user location, for more details please refer to Apple’s official docs
Fetch a route
How to fetch a route
Start navigation
Once we have fetched routes, we need to construct a NavigationOptions to build a NavigationViewController, and then call UIViewController’s present function to display NavigationView.
And there we go:
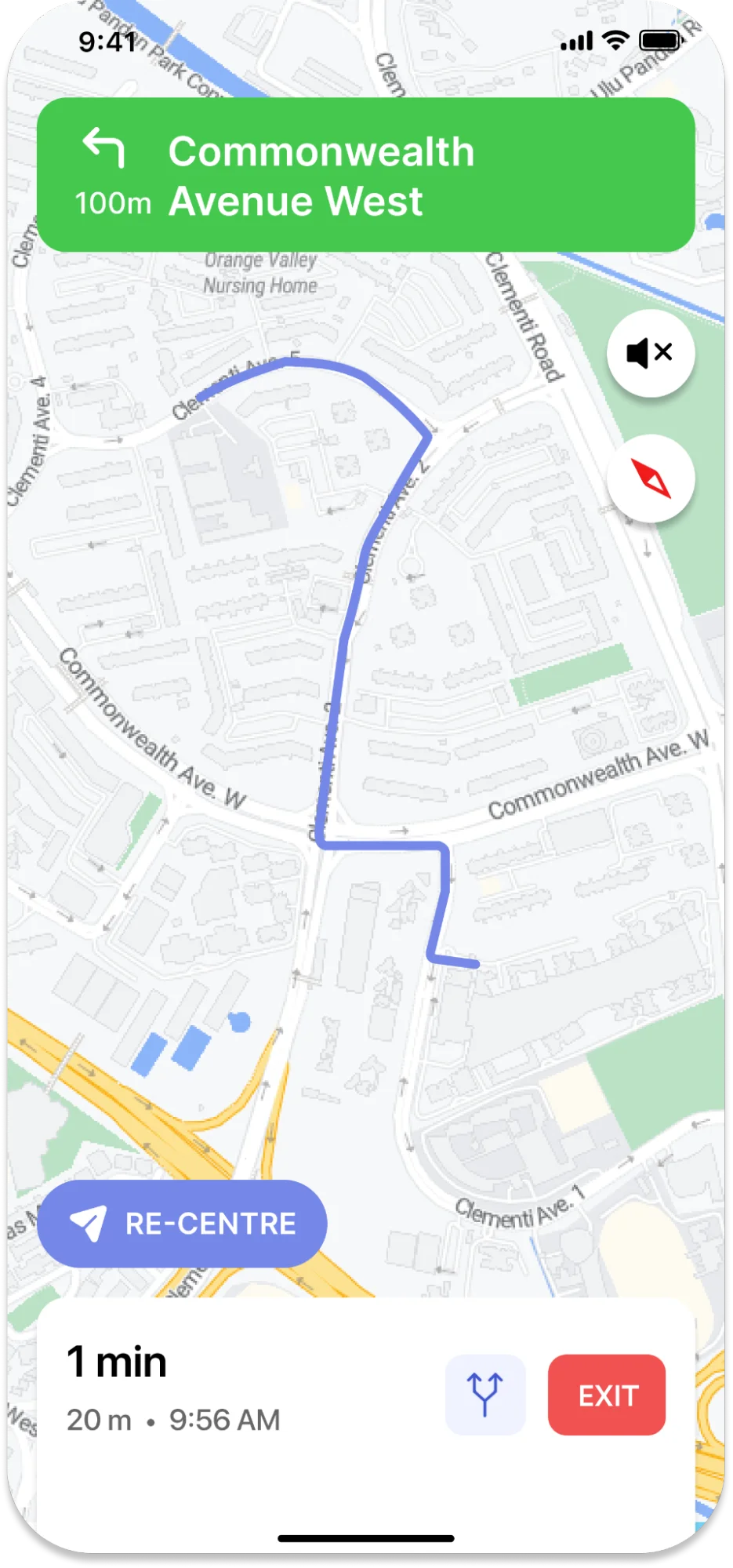