Custom Waypoint Styling
Easily tailor the waypoint styling to suit your preferences and create a visually appealing and intuitive navigation experience.
This example shows:
-
How to customize the UI of waypoints displayed in a route
-
The waypoint styling is customized in CustomWaypointStylingViewController
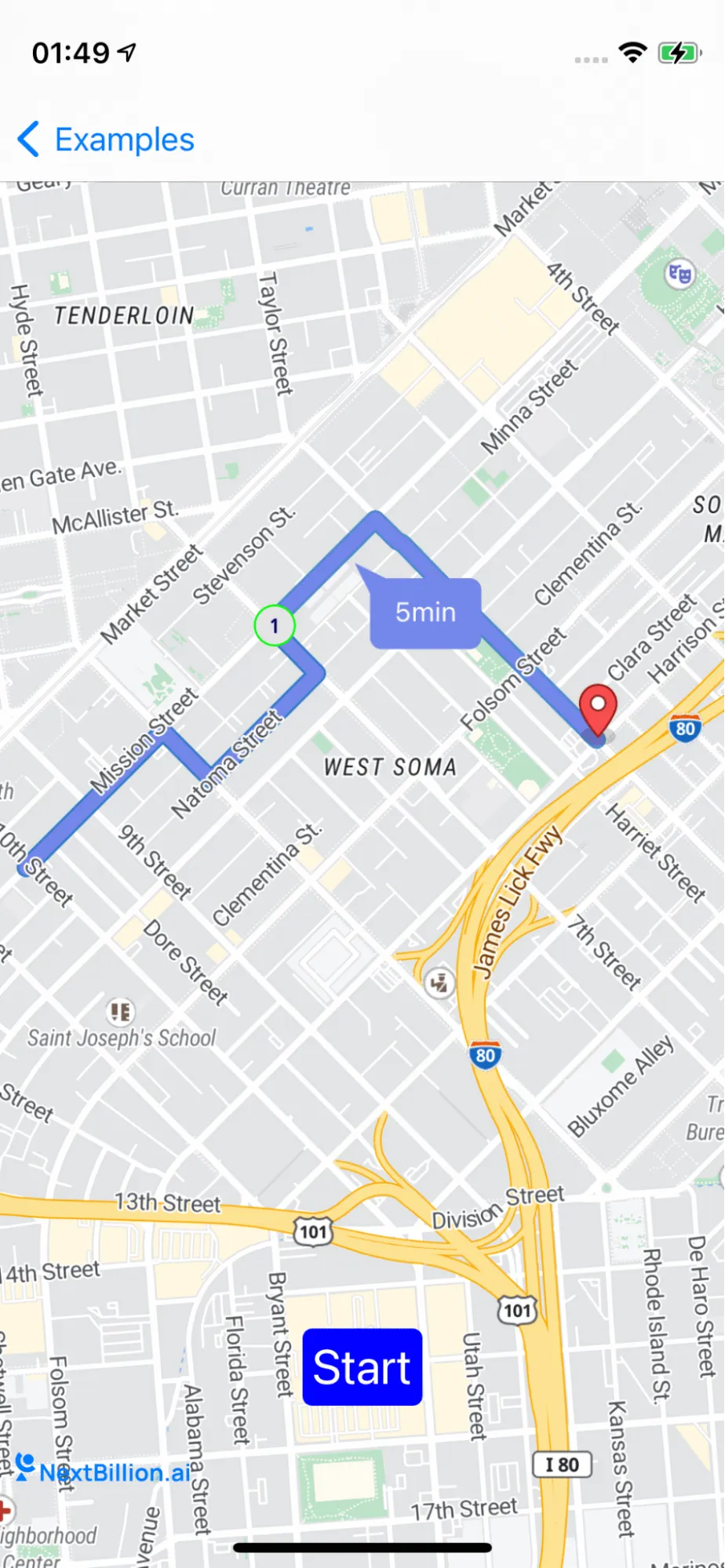
For all code examples, refer to Navigation Code Examples
CustomWaypointStylingViewController view source
The code example is for a CustomWaypointStylingViewController class. This class is used to customize the UI of waypoints displayed in a route. The class does this by overriding the navigationMapView(_:waypointStyleLayerWithIdentifier:source:), navigationMapView**(:waypointSymbolStyleLayerWithIdentifier:source:)**, and **navigationMapView(:shapeFor:legIndex:)** methods. The navigationMapView(_:waypointStyleLayerWithIdentifier:source:) method is used to customize the style of the waypoint circle. The method takes three parameters:
- mapView: The NavigationMapView instance that is displaying the route.
- identifier: The identifier of the style layer.
- source: The source of the style layer.
The method returns a NGLStyleLayer instance that represents the custom style of the waypoint circle. The NGLStyleLayer instance can be used to specify the color, opacity, radius, and stroke of the waypoint circle.
The navigationMapView(_:waypointSymbolStyleLayerWithIdentifier:source:) method is used to customize the style of the waypoint symbol. The method takes three parameters:
-
mapView: The NavigationMapView instance that is displaying the route.
-
identifier: The identifier of the style layer.
-
source: The source of the style layer.
The method returns a NGLStyleLayer instance that represents the custom style of the waypoint symbol. The NGLStyleLayer instance can be used to specify the text, opacity, font size, halo width, and halo color of the waypoint symbol.
The navigationMapView(_:shapeFor:legIndex:) method is used to customize the shape of the waypoints. The method takes three parameters:
-
mapView: The NavigationMapView instance that is displaying the route.
-
waypoints: The waypoints that are being displayed.
-
egIndex: The index of the current leg.
The method returns a NGLShape instance that represents the custom shape of the waypoints. The NGLShape instance can be used to specify the coordinates of the waypoints.
By overriding these methods, you can customize the UI of waypoints displayed in a route. Here are some examples of how you can use the CustomWaypointStylingViewController class to customize the UI of waypoints:
-
You can change the color of the waypoint circle.
-
You can change the opacity of the waypoint circle.
-
You can change the radius of the waypoint circle.
-
You can change the stroke of the waypoint circle.
-
You can change the text of the waypoint symbol.
-
You can change the opacity of the waypoint symbol.
-
You can change the font size of the waypoint symbol.
-
You can change the halo width of the waypoint symbol.
-
You can change the halo color of the waypoint symbol.
-
You can change the coordinates of the waypoints.