Custom Waypoint arrival screen
Customize the arrival experience to enhance user engagement and control during their navigation journey.
This example shows:
-
How to customize the arrival screen when arriving at a waypoint of the route
-
In the example code, arrival screen will show the waypoint name and user could choose to continue navigating to next waypoint
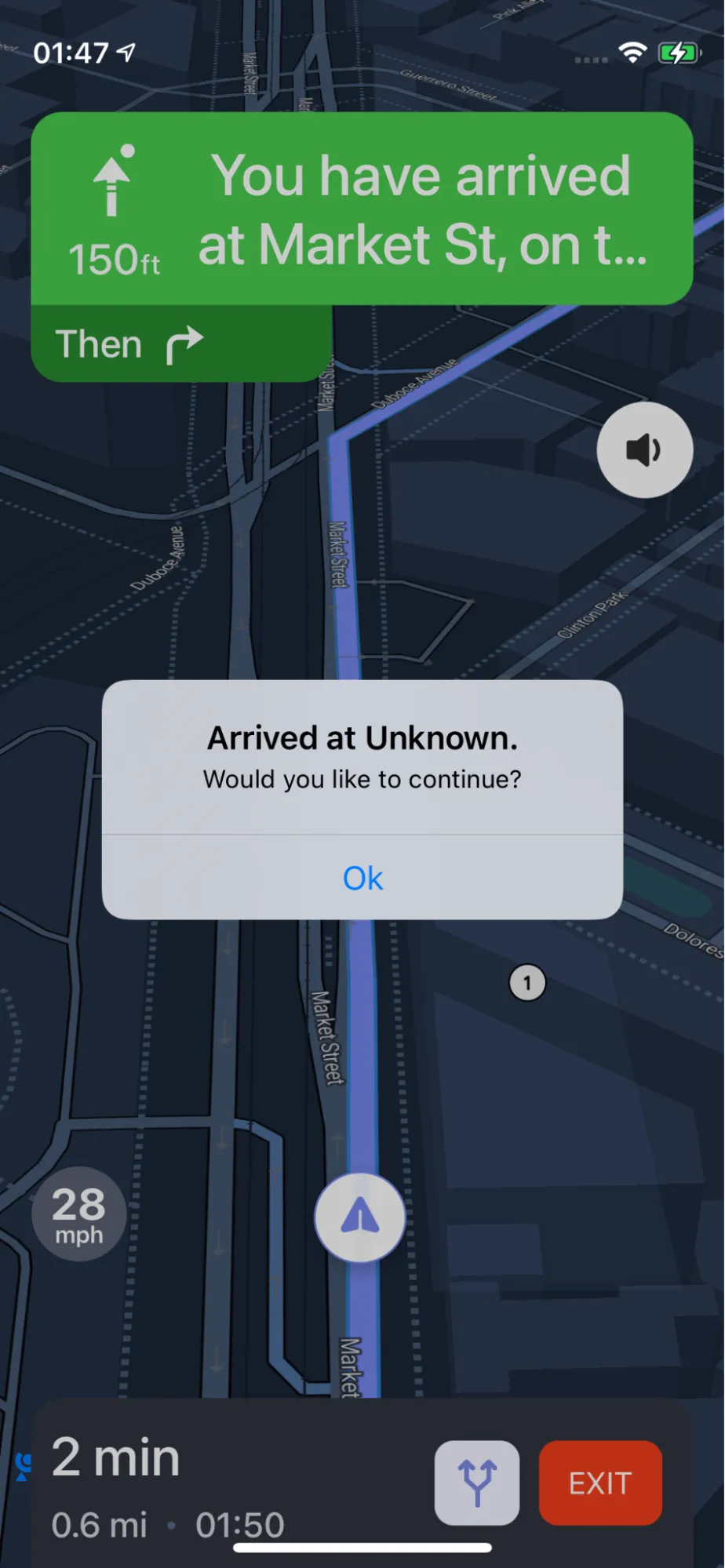
For all code examples, refer to Navigation Code Examples
CustomWaypointScreenController view source
This class is a view controller that allows users to navigate to a destination with waypoints. The class has the following properties:
-
origin: The origin location of the navigation.
-
waypoint: The waypoint location of the navigation.
-
destination: The destination location of the navigation.
-
routes: An array of Route objects that represent the routes between the origin, waypoint, and destination.
-
navigationService: A NBNavigationService object that is used to control the navigation.
-
navigationViewController: A NavigationViewController object that is used to display the navigation.
The class has the following methods:
-
viewDidLoad(): This method is called when the view controller is loaded. In this method, the navigation service is created and configured.
-
didArriveAtWaypoint(): This method is called when the navigation service arrives at a waypoint. In this method, an alert is presented to the user asking if they would like to continue to the next waypoint.
The code you provided also has an extension:
- CustomWaypointScreenController: NavigationViewControllerDelegate
This extension conforms the CustomWaypointScreenController class to the NavigationViewControllerDelegate protocol. This protocol allows the CustomWaypointScreenController class to respond to events from the navigation controller.
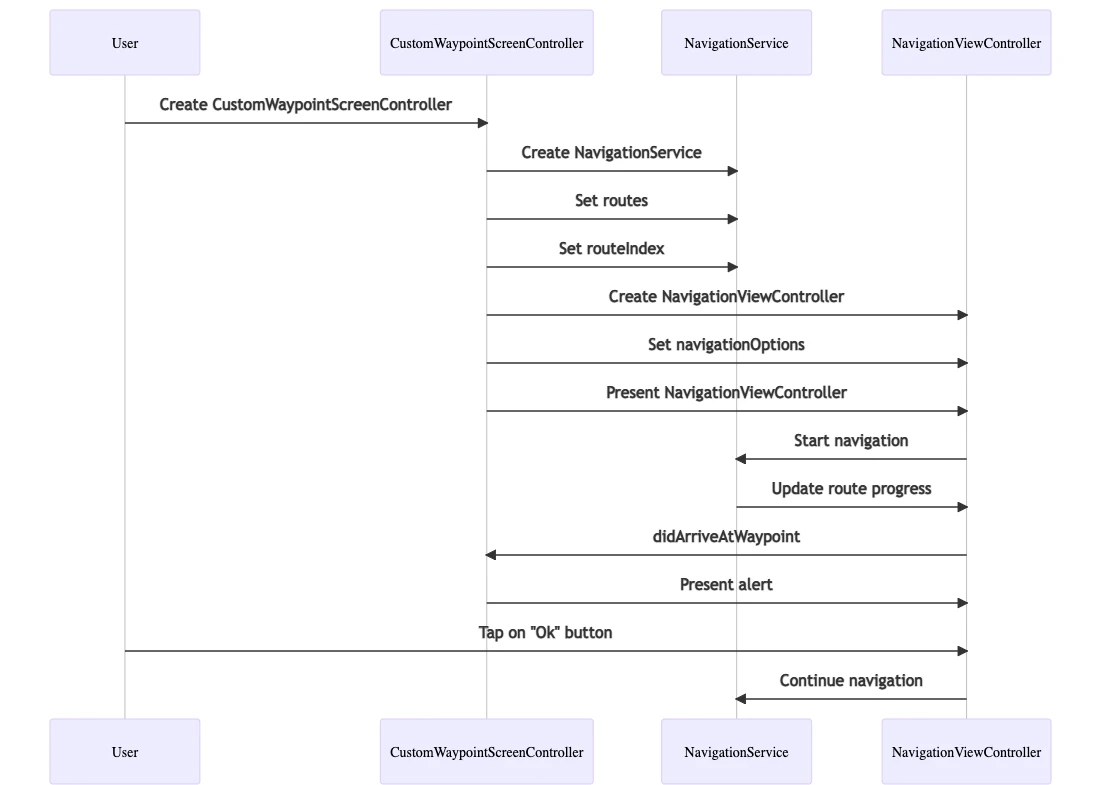