Custom Location Source
This example shows how to customize your location data source for NGLMapView.
- Custom your location data source for NGLMapView
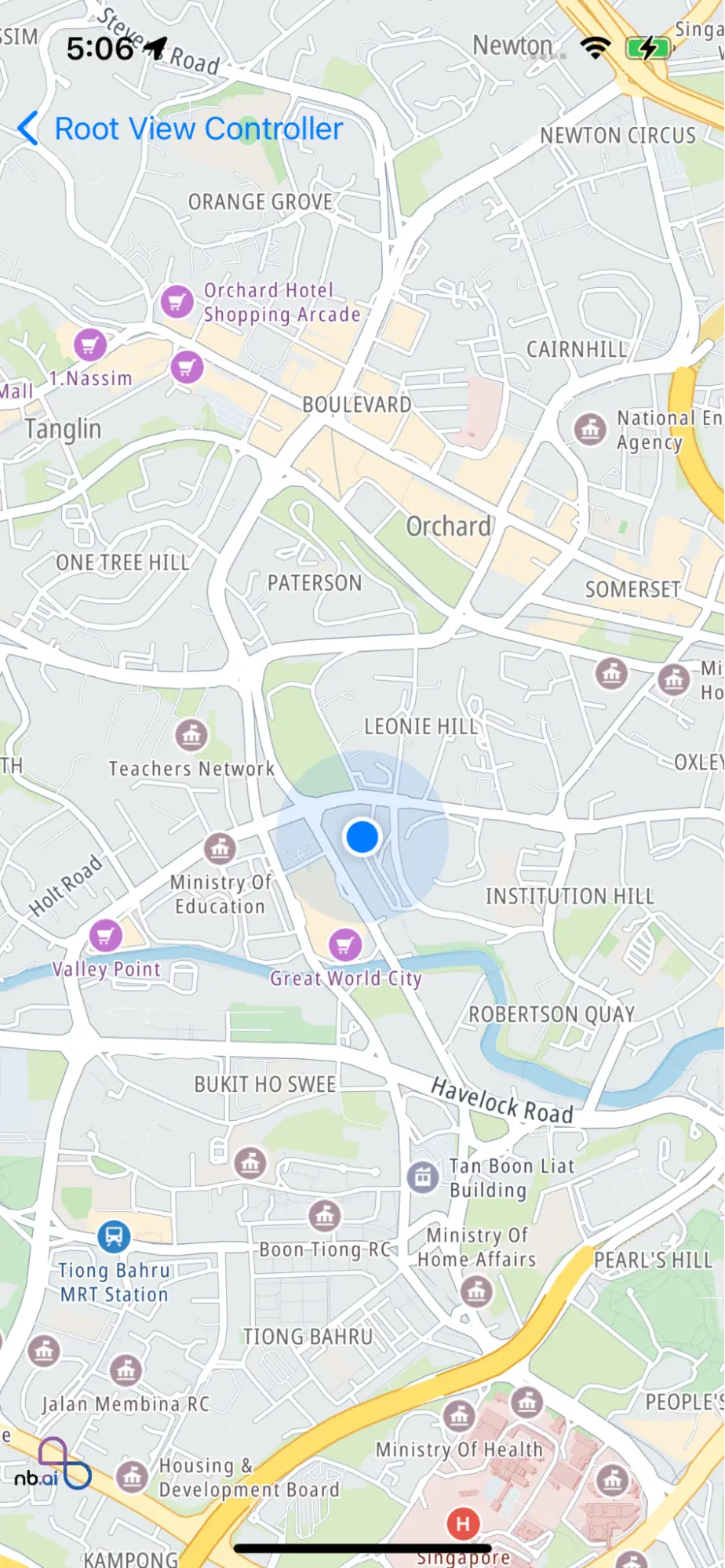
For all code examples, refer to Maps Code Examples
CustomLocationSourceViewController view source
CustomMapLocationManager
The example code implements a custom location source (CustomLocationSourceViewController) along with a custom location manager (CustomMapLocationManager).
CustomLocationSourceViewController is a subclass of UIViewController responsible for displaying a map view and setting up a custom location source
-
Initializing a map view (NGLMapView) and setting its frame to match the bounds of the current view.
-
Adding the map view as a subview to the current view.
-
Customizing the location source by assigning an instance of CustomMapLocationManager to the locationManager property of the map view.
-
Setting showsUserLocation to true to display the user's location on the map.
-
Setting userTrackingMode to .follow to track the user's location.
CustomMapLocationManager is a custom location manager that conforms to the NGLLocationManager protocol. It utilizes a CLLocationManager as its private property and sets itself as the delegate.
-
It implements the NGLLocationManagerDelegate protocol by setting the locationManager delegate to itself.
-
Various properties and methods of the location manager are mapped to the private locationManager instance using the delegate pattern.
-
It implements methods from the CLLocationManagerDelegate protocol and forwards these methods to the delegate object.
Summary: The provided code establishes a custom location source by utilizing a custom location manager. By setting the custom location manager, it is possible to replace the default location manager and achieve more flexibility and customization in handling location-related updates and delegate callbacks.