Query Features in box
This example shows how to Query features in the box on MapView
-
Query features about existing layer id and filter
-
Query features about custom layer id
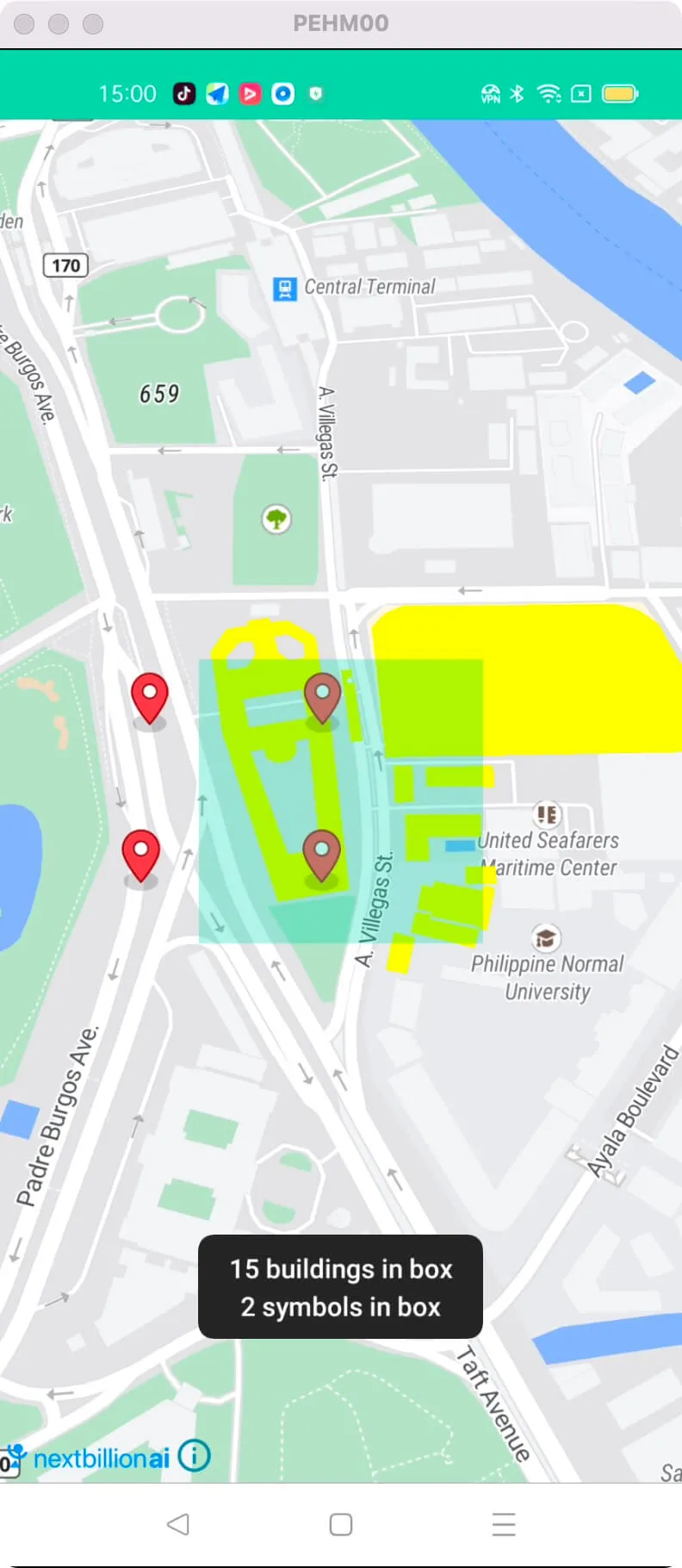
For all code examples, refer to Android Maps SDK Code Examples
activity_query_feature_and_symbol.xml view source
QueryFeatureAndSymbolActivity view source
The given code is an Android activity that demonstrates how to initialize a MapView, query features on a map, and display the results.
Initializing the MapView:
-
The onCreate method initializes the MapView by setting the content view and obtaining a reference to it using findViewById.
-
The onCreate method also calls mapView.onCreate(savedInstanceState) to create the MapView and mapView.getMapAsync(this) to get a reference to the NextbillionMap instance when it is ready.
Querying Features of an Existing Layer:
-
The code sets up a map and adds a layer called "highlighted-shapes-layer" with a source named "highlighted-shapes-source".
-
The selectionBox view is used to define a rectangular area on the map.
-
When the selectionBox is clicked, the queryRenderedFeatures method is called to query the features within the defined area.
-
The queried features are filtered based on a condition (in this case, the height is less than 10) using an Expression.
-
The queried features are displayed in a toast message.
Querying Features of a Custom Layer:
-
The code adds a custom symbol layer to the map, which is based on a GeoJSON source named "symbols-source".
-
The addSymbolLayer method reads GeoJSON data from a resource file and adds it as a source and layer to the map style.
-
The queryRenderedFeatures method is used to query the features within the defined area for the "symbols-layer" specifically.
-
The queried features are displayed in a toast message.
Other Lifecycle Methods: The code also includes various lifecycle methods (onStart, onResume, onPause, onStop, onSaveInstanceState, onDestroy, onLowMemory) that should be implemented when using the MapView to properly manage its lifecycle and handle configuration changes.
Note: The code uses the Nextbillion Maps SDK and NextbillionMap class to handle map-related operations. It also utilizes the Style class to define the map's visual style, GeoJsonSource class to add data sources, and various layer classes to display map features.