MapView Polyline
This example shows how to add PolyLines in MapView
-
Add Polyline from a set of Latlng
-
Set Polyline stroke
-
Set polyline colour
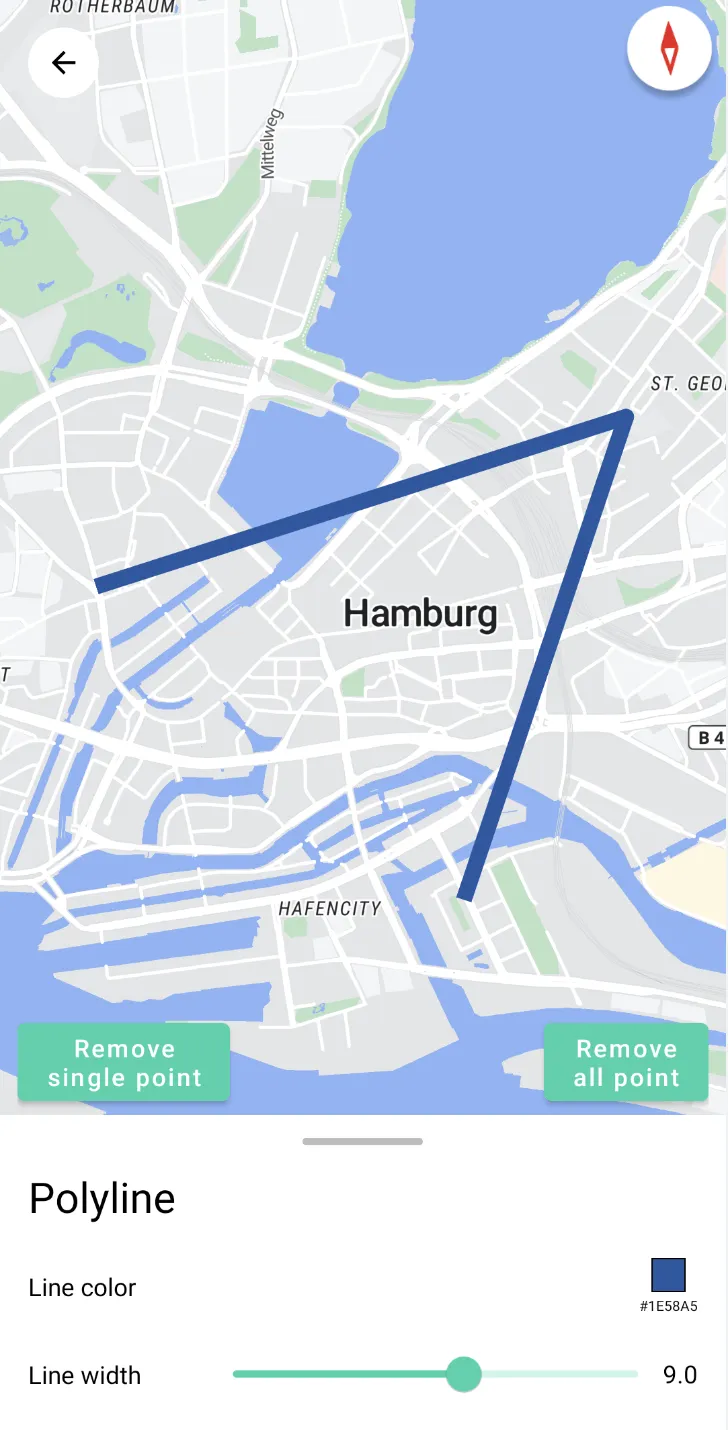
For all code examples, refer to Android Maps SDK Code Examples
activity_polyline.xml view source
PolylineActivity view source
-
applyPolyline(latLng: LatLng)
-
This method is used to apply a polyline on the map.
-
It takes a LatLng object as a parameter, representing a point on the polyline.
-
First, the point is added to the points list.
-
If the size of the points list is less than 2, it means there are not enough points to draw a polyline, so a marker is added to the map.
-
If the size of the points list is greater than or equal to 2, it means there are enough points to draw a polyline, so the previous polyline is removed and a new one is created.
-
Finally, the updateFloatButtonStatus method is called to update the status of the floating button.
-
-
removeSingleLine
-
This method is used to remove the last added point, thereby deleting the last segment of the polyline.
-
If the size of the points list is less than or equal to 2, it means there are not enough points to draw a polyline, so the removeAllPolyline method is called to remove all polylines and return.
-
If the size of the points list is greater than 2, the last point is removed, and a new polyline is created.
-
Finally, the updateFloatButtonStatus method is called to update the status of the floating button.
-
-
recreatePolyline
-
This method is used to recreate the polyline.
-
First, the previous polyline (if it exists) is removed.
-
A new polyline is created based on the points in the points list and assigned to the polyline variable.
-
The color of the polyline is set to the color specified by the lineColor variable.
-
The width of the polyline is set to the width specified by the lineWidth variable.
-
The animateBound method is called to fit the polyline's position.
-
-
removeAllPolyline
-
This method is used to remove all polylines.
-
The points list is cleared.
-
All markers and polylines on the map are cleared.
-
The polyline variable is set to null.
-
The updateFloatButtonStatus method is called to update the status of the floating button.
-
-
animateBound
-
This method is used to adjust the map's display area based on the position of the polyline.
-
If the size of the points list is less than 2, it means there are not enough points to draw a polyline, so it returns directly.
-
A LatLngBounds object is created based on the points in the points list.
-
The animateCameraBox method is called to animate the map's display area.
-
-
animateCameraBox
-
This method is used to animate the map's display area to include the specified boundary range.
-
A CameraPosition object is created based on the boundary range and padding.
-
If the CameraPosition object is not null, a CameraUpdate object is created, and the animateCamera method is used to animate the map's display area.
-
The code also includes various lifecycle methods (onStart, onResume, onPause, onStop, onSaveInstanceState, onDestroy, onLowMemory) that should be implemented when using the MapView to properly manage its lifecycle and handle configuration changes.
Note: The code uses the Nextbillion Maps SDK, which provides map-related functionalities. It also utilizes the LatLng class to represent geography.