Animate Image Source Layer
This example shows how to Animate the Runtime Map style layer
- Add Image Source layer
- Update the Image source every second
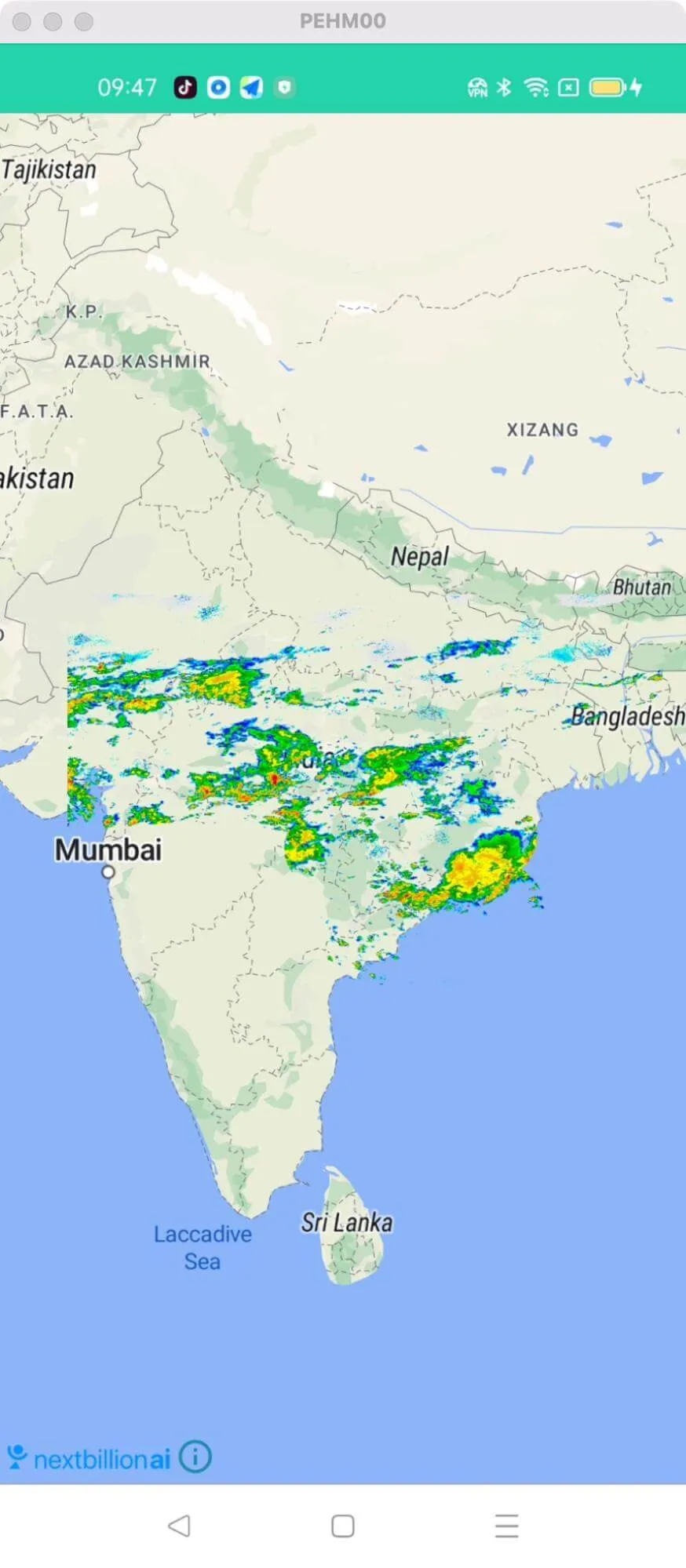
For all code examples, refer to Android Maps Code Examples
activity_animated_image_source.xml view source
AnimatedImageSourceActivity view source
This example demonstrates how to create an animation using a series of images with an ImageSource in the NextbillionMap library. The animation consists of a radar image that changes every second.
Initializing MapView:
- The MapView is initialized in the onCreate method by finding the view with the ID mapView from the layout file.
- The onCreate method also calls mapView.onCreate(savedInstanceState) to create the MapView and mapView.getMapAsync(this) to get a reference to the NextbillionMap instance when it is ready.
Adding Image Source Layer:
- The onMapReady method is called when the NextbillionMap instance is ready.
- A LatLngQuad is created to define the corners of a quadrilateral region on the map.
- A CameraPosition is defined to set the initial camera position of the map.
- An ImageSource is created with an ID, the LatLngQuad, and the resource ID of the first radar image.
- A RasterLayer is created with an ID and the ID of the ImageSource.
- The map style is set using a Style.Builder by specifying the style JSON URI, adding the ImageSource, and adding the RasterLayer.
- A RefreshImageRunnable is created to handle the animation by periodically changing the image of the ImageSource.
- The RefreshImageRunnable is scheduled to run every 100 milliseconds.
Animating Image Source:
- The RefreshImageRunnable class is a custom Runnable implementation.
- It keeps track of the current image index and an array of Bitmaps representing the radar images.
- In the constructor, it initializes the array of Bitmaps with the radar images and sets the initial image index.
- In the run method, it updates the image of the ImageSource with the current Bitmap.
- The image index is incremented, and if it exceeds the number of images, it is reset to 0.
- The RefreshImageRunnable is rescheduled to run after 1 second (1000 milliseconds) using the Handler instance.
Additional notes:
- The code includes lifecycle methods (onStart, onResume, onPause, onStop, onDestroy, onSaveInstanceState) to manage the lifecycle of the MapView.
- The code uses Nextbillion map library classes and methods for map-related operations.
- The code also includes utility methods (getBitmap) to convert resource IDs to Bitmaps for the radar images.