Custom destination marker
Enhance the visual representation of destination markers and provide users with real-time ETA information.
This example shows:
-
How to Custom destination marker
-
How to show route ETA on top of route line on map
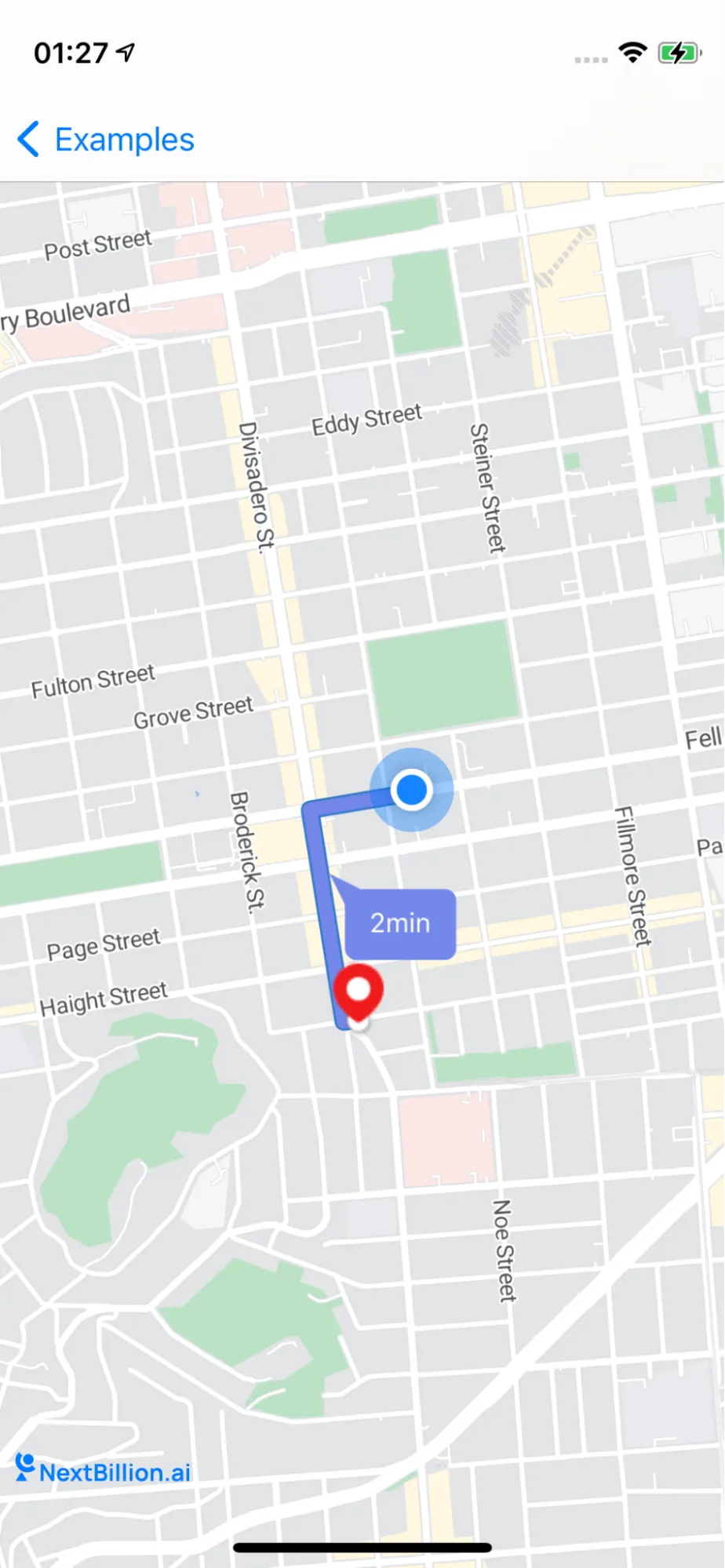
For all code examples, refer to Navigation Code Examples
CustomDestinationMarkerController view source
The class CustomDestinationMarkerController is a view controller that allows users to add a custom destination marker to a map. The class has the following properties:
-
mapView: A NavigationMapView object that displays the map.
-
routes: An array of Route objects that represent the routes between the user's current location and the custom destination marker.
The class has the following methods:
-
viewDidLoad(): This method is called when the view controller is loaded. In this method, the map view is created and configured.
-
didLongPress(): This method is called when the user long-presses on the map. In this method, a custom destination marker is added to the map at the location of the long press.
-
startNavigation(): This method is called when the user taps the "Start Navigation" button. In this method, a navigation controller is created and presented to the user. The navigation controller uses the routes property to display the route between the user's current location and the custom destination marker.
-
addNewDestinationcon(): This method adds a new destination annotation to the map.
-
requestRoutes(): This method requests routes between the user's current location and the custom destination marker.
-
mapView(_:imageFor:): This method is called by the map view to get an image for an annotation. In this method, a custom image is returned for the destination annotation.
-
navigationViewController(_:imageFor:): This method is called by the navigation controller to get an image for an annotation. In this method, a custom image is returned for the destination annotation.
The code also has two extensions:
-
CustomDestinationMarkerController: NGLMapViewDelegate
-
CustomDestinationMarkerController: NavigationViewControllerDelegate
These extensions conform the CustomDestinationMarkerController class to the NGLMapViewDelegate and NavigationViewControllerDelegate protocols. These protocols allow the CustomDestinationMarkerController class to respond to events from the map view and the navigation controller.