Track current location on NavNextbillionMap
This example shows how to request and update user location on NavNextbillionMap.
-
Activity will get and display the user's location on a map once the map is loaded successfully
-
A floating button is provided on the screen, each time user clicks on the button, the camera will focus and zoom to the user's current location
In this example we do not handle location permission requests, you can refer to the official guide for assistance: https://developer.android.com/training/location/permissions
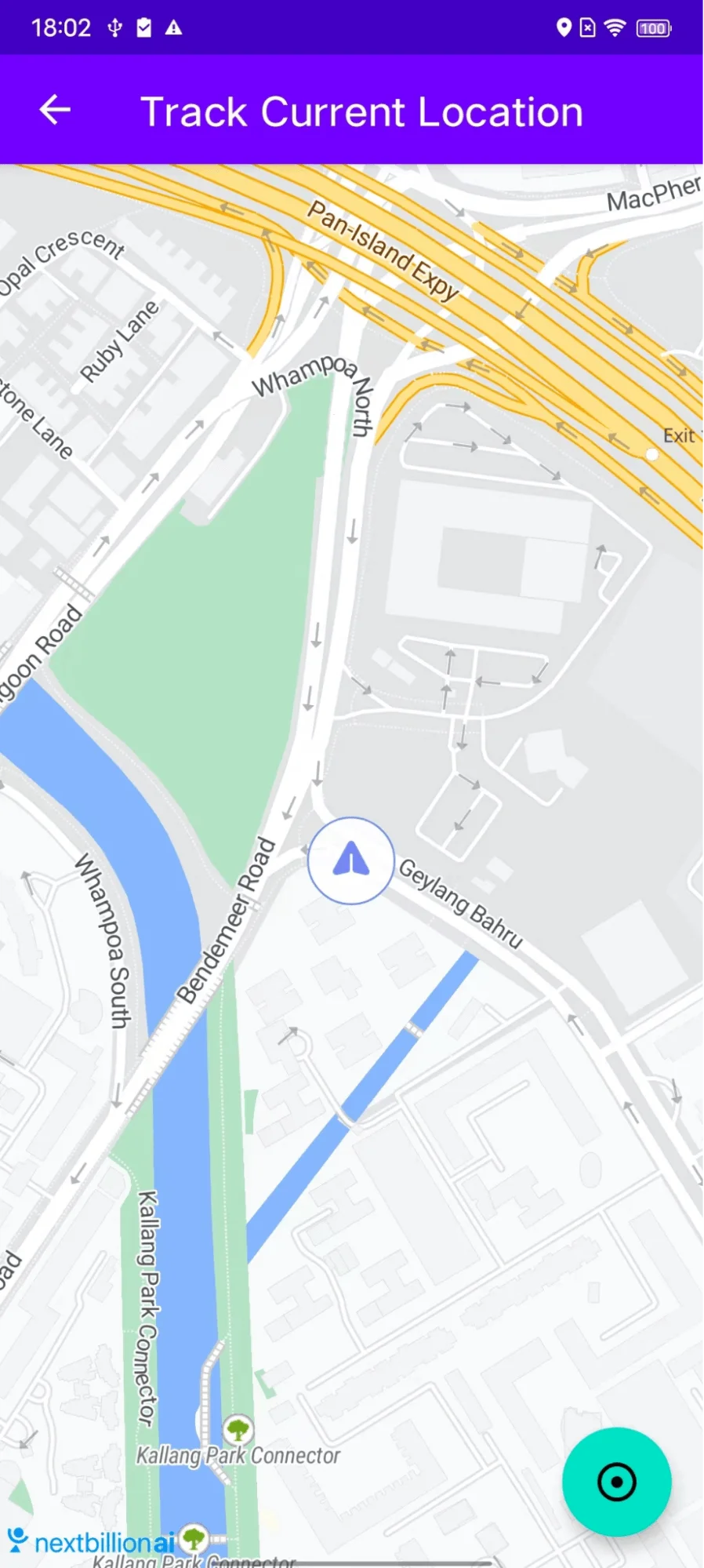
For all code examples, refer to Navigation Code Examples
activity_track_current_location.xml view source
TrackCurrentLocationActivity view source
Code Highlights
-
The mapView.getMapAsync() function will trigger the onMapReady() override function, and we set the map style and mode here.
-
The location engine initialization is also done in the onMapReady() function, it will try to get the device's last known location, then use nbMap.updateLocation() to show the location info on map
-
The code example also uses a float button to adjust the camera position and zoom level to provide a better visual experience to the user.
Code summary
The code snippet is for an Android activity that tracks the current location of the user and displays it on a map. The activity first creates a MapView object and sets its onCreate() listener. The onCreate() listener then gets the map's getStyle() and uses it to create a NavNextbillionMap object. The NavNextbillionMap object is then used to update the map's location layer render mode to RenderMode.GPS.
The activity then creates a LocationEngine object and requests location updates from it. The location updates are received by the TrackCurrentLocationCallback object, which updates the map's location with the latest location information.
The activity also has a FloatingActionButton object that is used to focus the camera on the user's current location. When the FloatingActionButton is clicked, the activity calls the animateCamera() method, which focuses the camera on the user's current location.
The activity implements the following lifecycle methods:
onStart(): This method is called when the activity starts. It calls the onStart() method of the MapView object.
onResume(): This method is called when the activity resumes. It calls the onResume() method of the MapView object and requests location updates from the LocationEngine object.
onLowMemory(): This method is called when the device is low on memory. It calls the onLowMemory() method of the MapView object.
onPause(): This method is called when the activity pauses. It calls the onPause() method of the MapView object and removes location updates from the LocationEngine object.
onStop(): This method is called when the activity stops. It calls the onStop() method of the MapView object.
onDestroy(): This method is called when the activity is destroyed. It calls the onDestroy() method of the MapView object.
The activity also uses the following constants:
CAMERA_ANIMATION_DURATION: This constant is the duration of the animation used to focus the camera on the user's current location.
DEFAULT_CAMERA_ZOOM: This constant is the default zoom level of the map.
UPDATE_INTERVAL_IN_MILLISECONDS: This constant is the interval at which the LocationEngine object requests location updates.
FASTEST_UPDATE_INTERVAL_IN_MILLISECONDS: This constant is the minimum interval at which the LocationEngine object requests location updates.
The activity uses the following classes:
-
MapView: This class represents a map view.
-
FloatingActionButton: This class represents a floating action button.
-
NextbillionMap: This class represents a Nextbillion map.
-
LocationEngine: This class provides access to location services.
-
LocationEngineRequest: This class represents a request for location updates.
-
LocationEngineResult: This class represents the result of a location update request.
-
TrackCurrentLocationCallback: This class implements the LocationEngineCallback interface and is used to receive location updates.