Customize Route Line Style
This example shows:
-
How to customize the styling of Route line
-
routeColor
-
routeScale
-
alternativeRouteScale
-
routeShieldColor
-
routeFloatDurationBackgroundPrimary
-
maneuverArrowColor
-
maneuverArrowBorderColor
-
routeWayNameBackGroundColor
-
routeWayNameTextColor
-
-
How to config custom navigation style using NavLauncherConfig.Builder
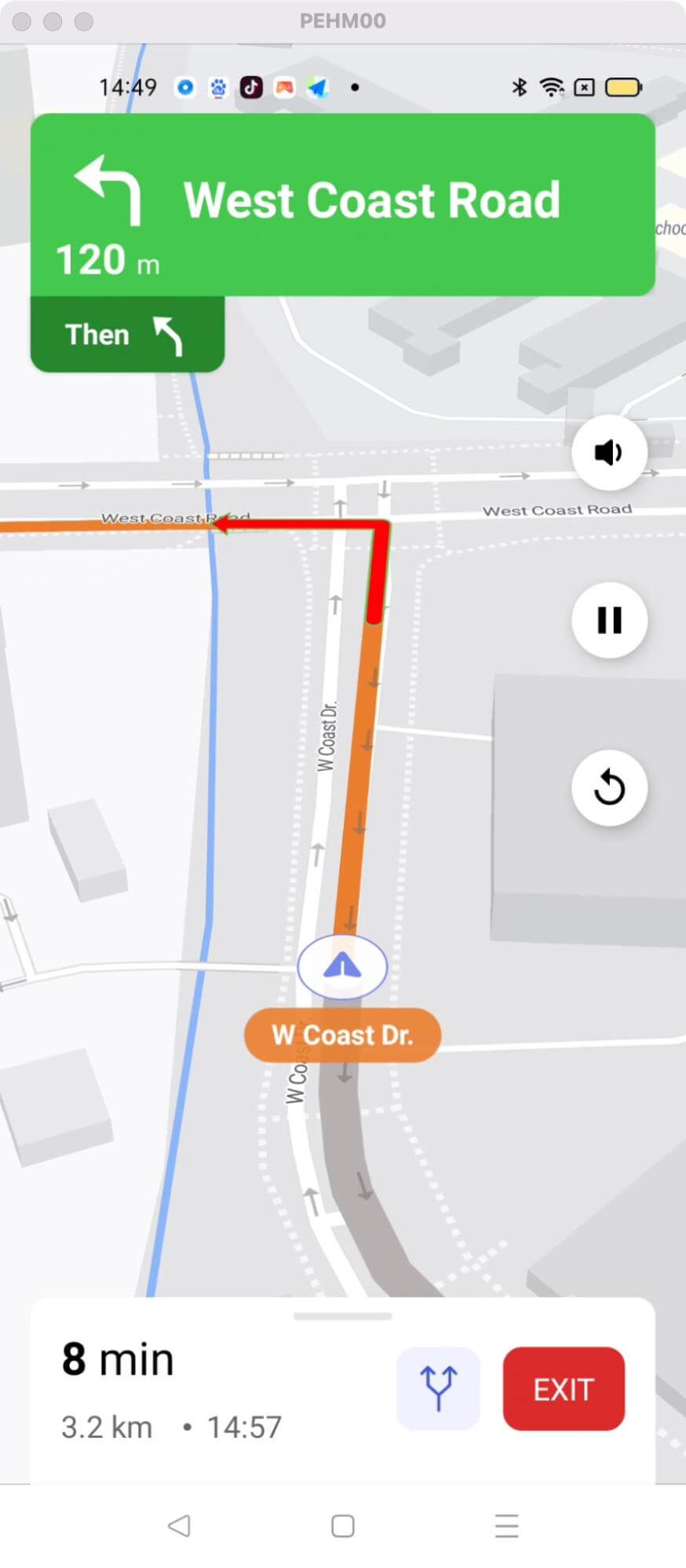
For all code examples, refer to Navigation Code Examples
activity_route_line_style.xml view source
themes.xml view source
CustomRouteLineStyleActivity view source
Code Highlights
This code example shows how to customize the navigation view route line style via themes.xml configuration file, the color of route line-related UI elements are defined in the file.
In the above theme file, we have customized some route line color in style with name CustomRouteStyle
, then we applied the CustomRouteStyle
in Theme.CustomNavigationRouteLine
for day style and Theme.CustomNavigationRouteLineDark
for night style. We can also do some further customizations in Theme.CustomNavigationRouteLine
and Theme.CustomNavigationRouteLineDark
based on needs (e.g. show different UI color during day/night).
In code we use configBuilder.lightThemeResId()
and configBuilder.darkThemeResId()
to specify the theme for the Navigation view in day style and night style respectively.
Code summary
This code example defined the theme styles Theme_CustomNavigationRouteLine based on NavigationViewLight and Theme_CustomNavigationRouteLineDark based on NavigationViewDark
The navViewRouteStyle item is set to @style/CustomRouteStyle. The CustomRouteStyle customizes the appearance of the navigation route line in the navigation view.
The following items are defined within CustomRouteStyle:
-
routeColor to specify the color of the route line
-
routeScale and alternativeRouteScale to determine the scale of the route line and alternative route lines respectively
-
routeShieldColor to set the color of the shields along the route
-
maneuverArrowColor and maneuverArrowBorderColor to define the colors of the maneuver arrows
-
routeWayNameBackGroundColor and routeWayNameTextColor to specify the background color and text color of the route way names.
configBuilder.lightThemeResId(R.style.Theme_CustomNavigationRouteLine)
sets the light theme for the navigation UI, using the Theme_CustomNavigationRouteLine style defined earlier. This style determines the appearance of the route line in the navigation view.
configBuilder.darkThemeResId(R.style.Theme_CustomNavigationRouteLineDark)
sets the dark theme for the navigation UI, using the Theme_CustomNavigationRouteLineDark style defined earlier. This style is applied when the device's dark mode is enabled.
Besides, the action after clicking the fetch route button shows how to fetch a route using NBNavigation.fetchRoute(origin, destination, new Callback<DirectionsResponse>() { ... });
The origin and destination points are passed as parameters, along with a callback to handle the response asynchronously.