Customize Navigation View UI elements
Navigation View is the UI provided to show the navigation route process. In this example we will show how to do customization on Navigation View, including:
-
Add a new UI element by customizing the activity layout
-
Show or hide existing Navigation View UI elements
As is shown in the above image, we customized the Navigation View app bar and added a switch button to it. A user tapping the switch button will change the visibility of two UI elements (Recenter Button and Sound button), which are originally existing in Navigation View.
For all code examples, refer to Navigation Code Examples
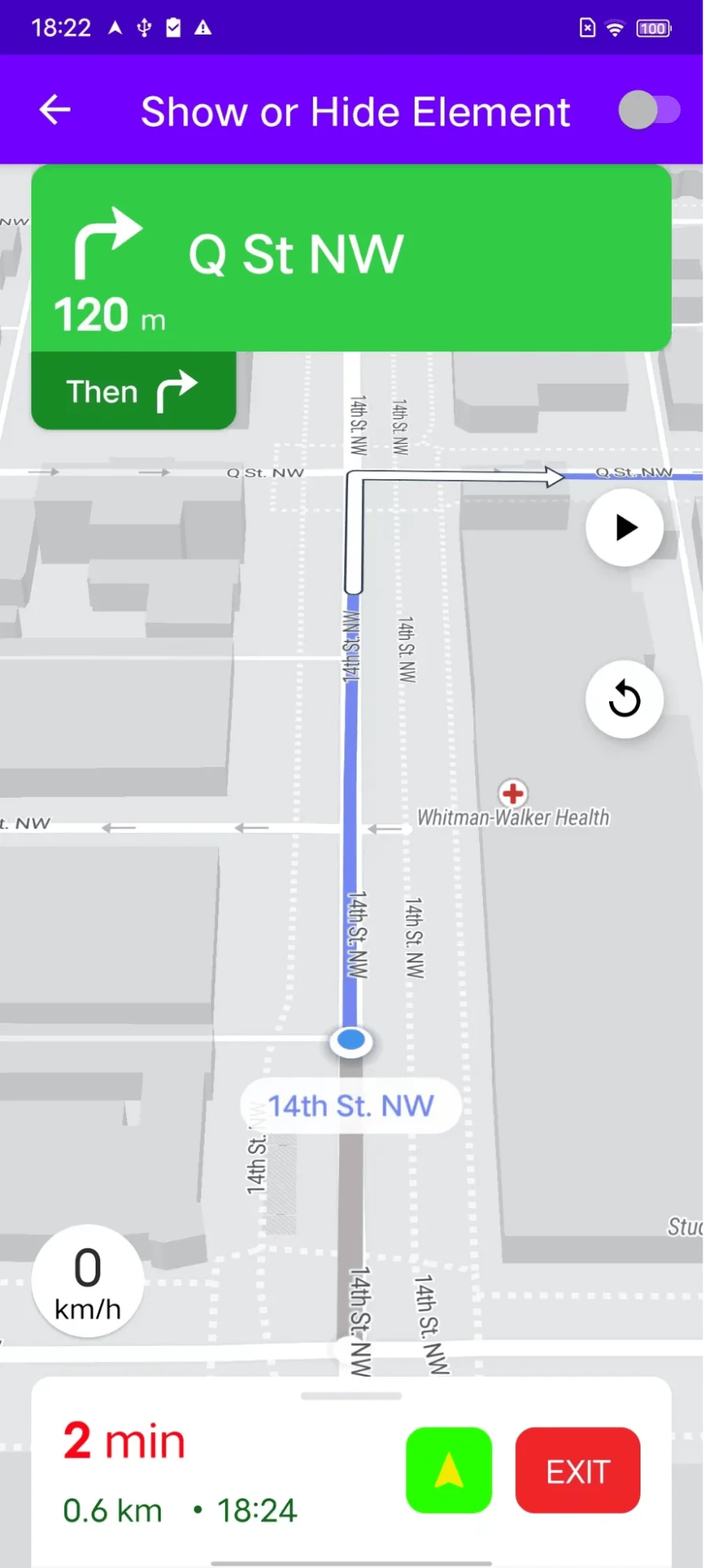
activity_custom_element_visibility.xml view source
CustomElementVisibilityActivity view source
Code Highlights
The main logic of the code example is to use a switch button to control the visibility of the Recenter button and the Sound button in the navigation view.
The controlDisPlayOfElement() function shows how to display or hide the corresponding view during navigation.
Code summary
The code snippet is for an Android activity that allows users to control the visibility of elements in a navigation view. The activity extends the AppCompatActivity class and implements the OnNavigationReadyCallback and NavigationListener interfaces.
The activity's onCreate() method first inflates the activity_custom_element_visibility layout. It then finds the navigationView view in the layout and calls its onCreate() method. Finally, it calls the initialize() method on the navigationView to initialize it.
The activity's onCreateOptionsMenu() method inflates the mainmenu menu. It then finds the myswitch menu item and gets its SwitchCompat view. The activity then calls the controlDisplayOfElements() method to set the initial visibility of the elements in the navigation view. The activity also sets a listener on the myswitch view so that it can be updated when the user changes the switch's state.
The controlDisplayOfElements() method takes a boolean value as input. If the value is true, the method shows the Recenter button and the Sound button in the navigation view. If the value is false, the method hides the buttons.
The onNavigationReady() method is called when the navigation view is ready. The method reads the original backend response from a file. It then modifies the response and creates a NavViewConfig object. The NavViewConfig object specifies the route that will be navigated, whether the speedometer will be shown and whether the navigation listener will be used. The method then calls the startNavigation() method on the navigation view to start navigation.
The convertStreamToString() method converts an input stream to a string. The method uses a Scanner object to read the stream and a delimiter to determine where the stream ends.
The loadJsonFromAsset() method loads a GeoJSON file from the assets folder. The method tries to open the file and read its contents. If the file cannot be opened, the method returns null.
The activity uses the NavigationView class to display a navigation view in the user interface. The activity also uses the OnNavigationReadyCallback and NavigationListener interfaces to interact with the navigation view.