Mapview Polyline
This example shows how to draw a polyline on the NGLMapView.
-
User long-press to select points.
-
Draw a Polyline between points.
-
Provide proxy methods to set properties of the Polyline, such as color and width. Also, provide some methods to handle user gesture events, such as selecting and deselecting the Polyline.
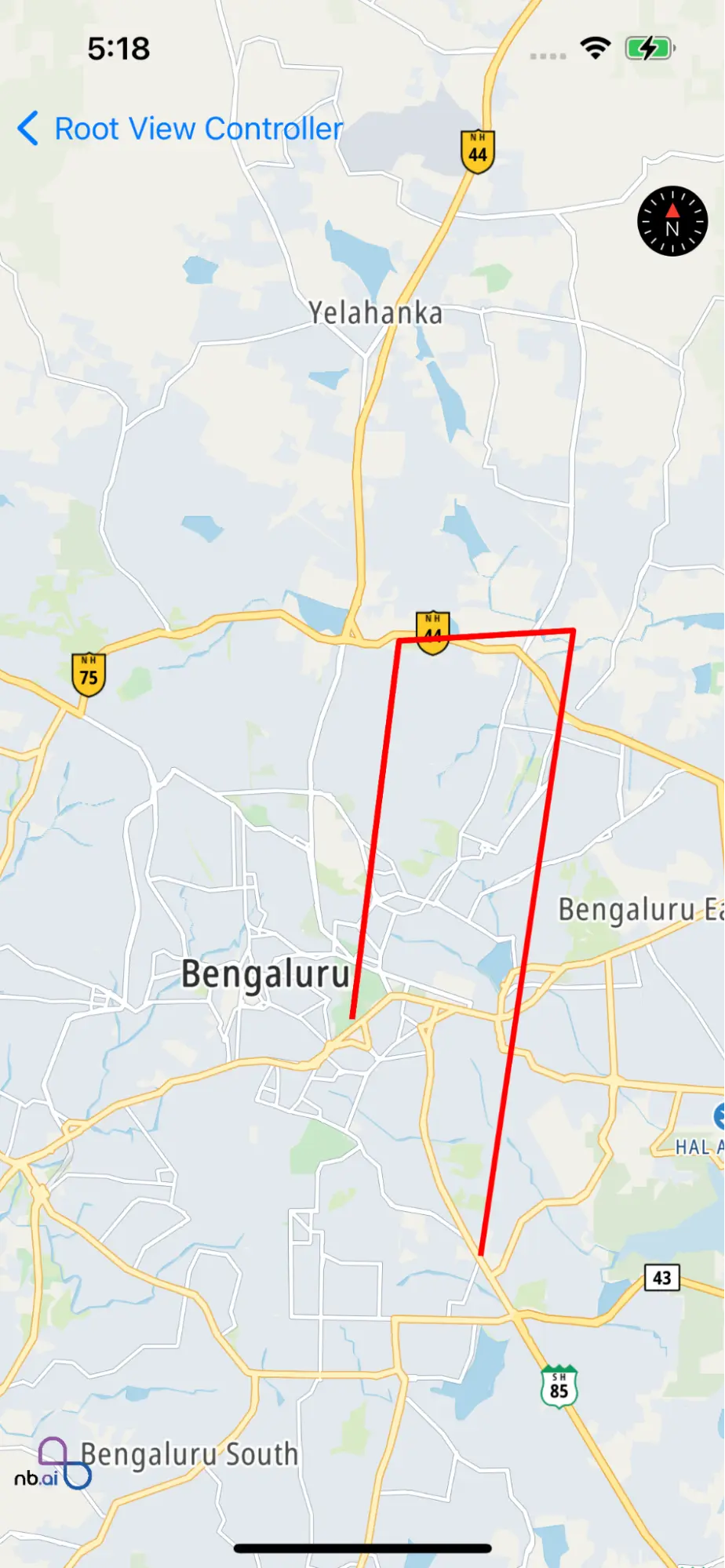
For all code examples, refer to Maps Code Examples
PolylineViewController view source
The example code sets up a view controller with a map view, allows drawing a polyline on the map by adding points via long-press gestures, and customizes the polyline's style and annotation events using the NGLMapViewDelegate methods.
Initialization of MapView
- The code initializes an instance of NGLMapView and assigns it to the nbMapView property. The map view is configured with a flexible width and height and added as a subview to the view controller's view. The map view's delegate is set to the view controller.
Drawing a Polyline
- The code defines a function named drawPolyline that creates a polyline on the map view. It checks if there are at least two points in the points array, creates an NGLPolyline object with the coordinates from the points array, and adds the polyline as an annotation to the map view.
Custom Polyline Style
-
The code includes several delegate methods from the NGLMapViewDelegate protocol to customize the style of the polyline and handle various events related to annotations.
-
mapView(_:didFinishLoading:) method sets the initial camera position.
-
mapView(_:alphaForShapeAnnotation:),mapView(_:strokeColorForShapeAnnotation:), mapView(_:fillColorForPolygonAnnotation:), mapView(_:lineWidthForPolylineAnnotation:) methods define the alpha value, stroke color, fill color, and line width for the polyline annotation, respectively.
-
mapView(_:didSelect:) and mapView(_:didDeselect:) methods handle the selection and deselection events of annotations and print their titles and subtitles.
Additionally, the code includes a long-press gesture recognizer that allows the user to add points to the polyline by long-pressing on the map view. The long-press gesture handler didLongPress retrieves the long-press location, converts it to a coordinate, adds the coordinate to the points array, and calls the drawPolyline function to update the polyline on the map.