MapView Polygon
This example shows how to draw a NGLPolygon on the NGLMapView.
-
Draw a Polygon by the given coordinates.
-
Provide proxy methods to set properties of the Polygon, such as color and alpha. Also, provide some methods to handle user gesture events, such as selecting and deselecting the Polyline.
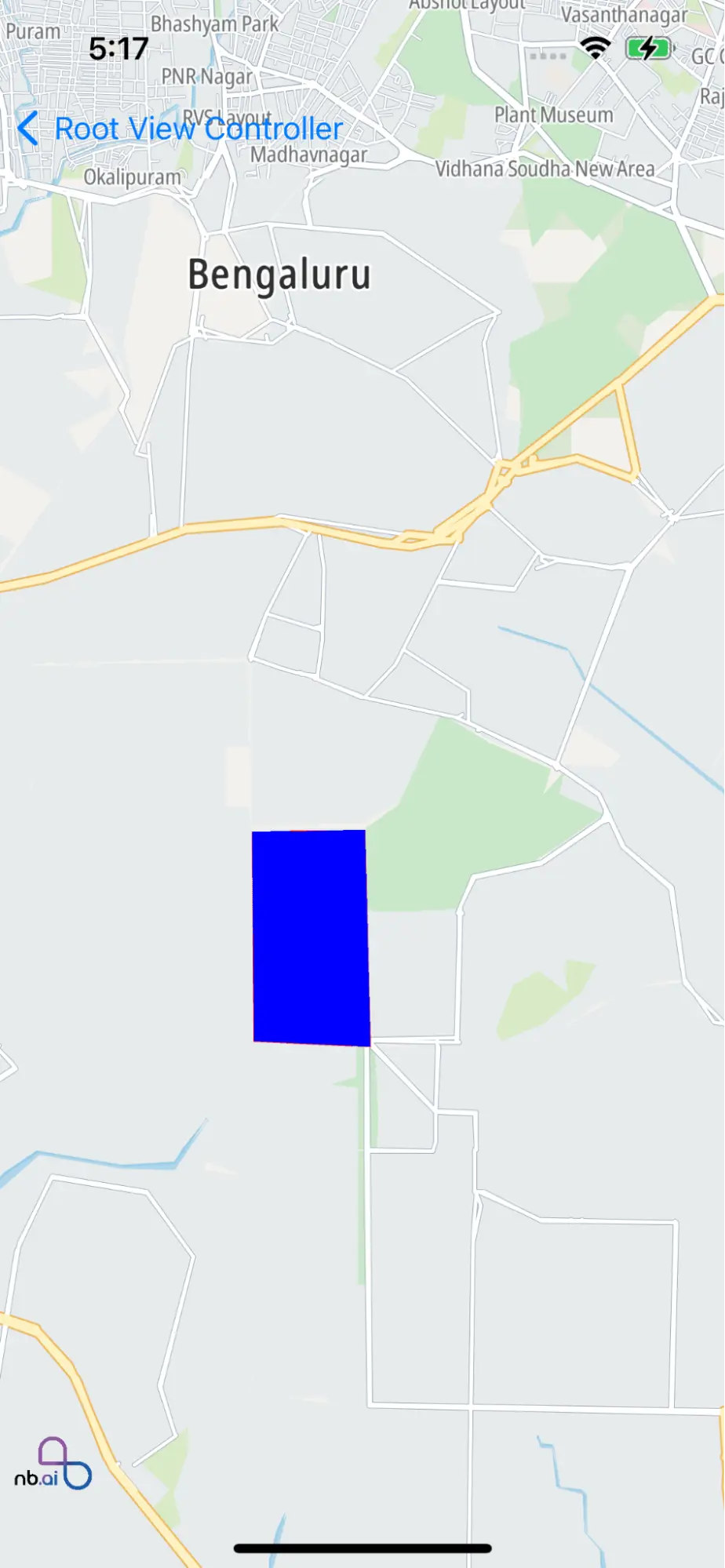
For all code examples, refer to Maps Code Examples
PolygonViewController view source
The example code sets up a view controller with a map view, allows drawing a polygon on the map, and customizes the polygon's style and annotation events using the NGLMapViewDelegate methods.
Initialization of MapView
- The code initializes an instance of NGLMapView and assigns it to the nbMapView property. The map view is configured with a flexible width and height and added as a subview to the view controller's view. The map view's delegate is set to the view controller.
Drawing a Polygon
- The code defines a function named drawPolygon that creates a polygon on the map view. It specifies an array of coordinates for the polygon's vertices and creates an NGLPolygon object with these coordinates. The existing annotations on the map view are removed, and the created polygon is added as an annotation to the map view.
Custom Polygon Style
-
The code includes several delegate methods from the NGLMapViewDelegate protocol to customize the style of the polygon and handle various events related to annotations.
-
mapView(_:didFinishLoading:) method sets the initial camera position.
-
mapView(_:alphaForShapeAnnotation:), mapView(_:strokeColorForShapeAnnotation:) mapView(_:fillColorForPolygonAnnotation:), mapView(_:lineWidthForPolylineAnnotation:) methods define the alpha value, stroke color, fill color, and line width for the polygon annotation, respectively.
-
mapView(_:didSelect:) and mapView(_:didDeselect:) methods handle the selection and deselection events of annotations and print their titles and subtitles.
-